Introduction
A vector is a versatile and widely used data structure in programming languages. It is an ordered collection of elements that can dynamically grow or shrink in size as needed. Vectors provide efficient random access to elements and support various operations such as insertion, deletion, and traversal. They are often implemented as arrays that automatically resize when the number of elements exceeds the current capacity. Vectors are useful for storing and manipulating sequences of data, making them valuable in many applications, including algorithms, data processing, and container classes. Their flexibility and efficiency make them a popular choice for managing dynamic collections of elements in programming languages.
Key Features of Vectors:
- Dynamic Size: Vectors can change in size during runtime, meaning elements can be added or removed without a fixed capacity.
- Contiguous Memory: Vectors store elements in contiguous memory locations, allowing for efficient element access through indexing.
- Automatic Resizing: When the vector reaches its capacity, it automatically resizes to accommodate additional elements, typically by doubling its size.
- Access and Modification: Elements in a vector can be accessed and modified using index-based notation, similar to regular arrays.
Example of using vectors in C++:

In this example, the vector “numbers” is dynamically resized as elements are added and removed. Vectors offer a powerful and convenient way to work with collections of varying sizes, making them a popular choice for many programming tasks.
Declaration and Type Checking
A vector is a dynamic data structure that allows for the efficient storage and manipulation of sequences of elements. When working with vectors, it is essential to understand the process of declaring and type checking them to ensure correct and reliable usage. This article will explore the declaration and type checking of vectors in programming languages.
I. Declaration of Vectors: To use a vector, it must be declared explicitly, indicating the data type of the elements it will hold. The declaration informs the compiler or interpreter about the structure and organization of the vector, allowing for appropriate memory allocation and optimization. The declaration typically includes the name of the vector and its declared data type.
Example:
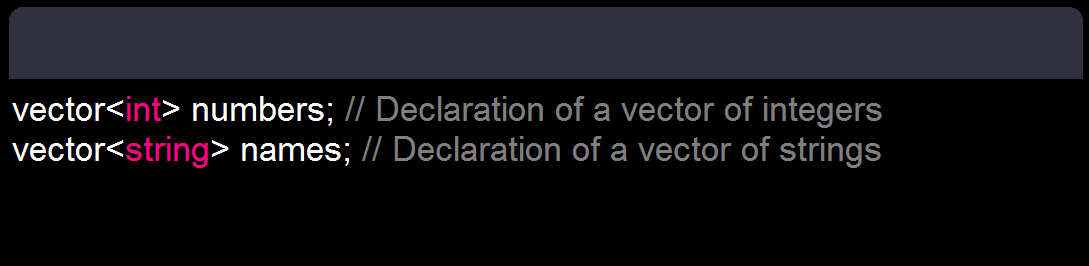
II. Specifying the Data Type: Each vector must be associated with a specific data type, which defines the kind of elements it can store. The data type can be any built-in or user-defined type, such as integers, floating-point numbers, characters, or even custom-defined classes. By specifying the data type, the vector ensures that only compatible elements can be stored.
III. Type Checking: Type checking is a crucial process that verifies the compatibility of elements being used with the declared data type of the vector. It ensures that only elements of the specified type are inserted into the vector and that operations performed on the vector adhere to the declared data type. Type checking can be performed statically during compilation or dynamically at runtime, depending on the programming language.
Static Type Checking: In statically typed languages, type checking occurs during compilation. The compiler analyzes the code and ensures that operations and assignments are consistent with the declared data type of the vector. If there is a type mismatch, the compiler raises a compile-time error, preventing the program from executing until the issue is resolved.
Dynamic Type Checking: In dynamically typed languages, type checking is performed at runtime. The interpreter checks the types of elements as they are being inserted or accessed within the vector. If a type mismatch is detected, the interpreter raises a runtime error, halting the program’s execution and providing an error message indicating the type inconsistency.
Type checking ensures the integrity and reliability of the vector by preventing incompatible data from being inserted or accessed. It minimizes the risk of type-related errors and enhances code robustness.
IV. Benefits of Declaration and Type Checking:
- Code Reliability: By explicitly declaring the data type and performing type checking, vectors ensure that the correct type of elements is used, minimizing the likelihood of runtime errors and improving the reliability of the program.
- Code Readability and Maintainability: Declaration and type checking enhance code readability by providing clear information about the expected data type for elements in the vector. It also improves code maintainability by enforcing consistency and reducing the chance of type-related bugs.
- Optimization Opportunities: Type information gained through declaration and type checking enables the compiler or interpreter to perform static analysis and optimization. This can lead to improved performance and memory utilization.
V. Handling Type Errors: When a type error occurs during the declaration or manipulation of a vector, appropriate error handling is necessary. The programming language may provide error messages or exceptions to alert programmers about type mismatches. It is essential to identify and correct type errors promptly to ensure the correctness and robustness of the program.
VI. Type Inference: In some programming languages, type inference allows the compiler or interpreter to automatically deduce the data type based on the initialization values of the vector. Type inference reduces the need for explicit type declarations and provides flexibility in coding while still ensuring type correctness.
Conclusion
In conclusion, the declaration and type checking of vectors are vital processes in programming languages. By explicitly declaring the vector’s data type and performing type checking, programmers ensure the correct usage and compatibility of elements within the vector. These processes enhance code reliability, readability, and maintainability. Proper declaration and type checking contribute to the creation of high-quality software systems, enabling programmers to work with confidence when utilizing vectors in their programs.
more related content on Principles of Programming Languages