Introduction
Variable size data structures, also known as dynamic data structures, are data structures in programming that can change in size during program execution. Unlike fixed-size data structures, which have a predetermined size that cannot be altered, variable-size data structures allow for flexibility in managing data.
Examples of variable-size data structures include dynamic arrays, linked lists, trees, and hash tables. These data structures are particularly useful when the number of elements to be stored is unknown or can change over time. They dynamically allocate memory as needed, enabling efficient memory utilization and minimizing wastage. Variable-size data structures are commonly used in various applications, such as handling input data of unknown size, managing collections with dynamic growth, and implementing dynamic memory management in programs.
I. Dynamic Arrays:
Dynamic arrays are a common example of variable-size data structures. They behave similarly to regular arrays but allow resizing as elements are added or removed. When a dynamic array reaches its capacity, it dynamically reallocates more memory, typically doubling its size, to accommodate additional elements. This resizing process ensures efficient memory usage while providing the ability to handle collections of various sizes.
Example:

II. Linked Lists:
Linked lists are another classic example of variable-size data structures. They consist of nodes, each containing data and a reference to the next node in the list. Linked lists can grow or shrink by adding or removing nodes, making them suitable for situations where the size of the data collection is not known in advance.
Example:
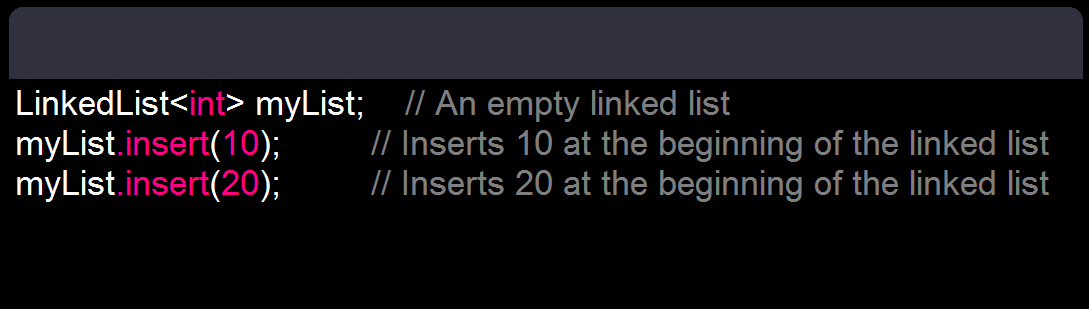
III. Trees:
Trees, such as binary search trees, are dynamic data structures that can grow or shrink as nodes are added or deleted. Trees are well-suited for efficient searching and retrieval of data, and their dynamic nature allows them to handle various amounts of data.
Example:

IV. Hash Tables:
Hash tables are dynamic data structures that store key-value pairs. They resize automatically to maintain an optimal load factor, ensuring efficient data retrieval and minimizing collisions. Hash tables are widely used in data storage and retrieval applications where key-based access is required.
Example:
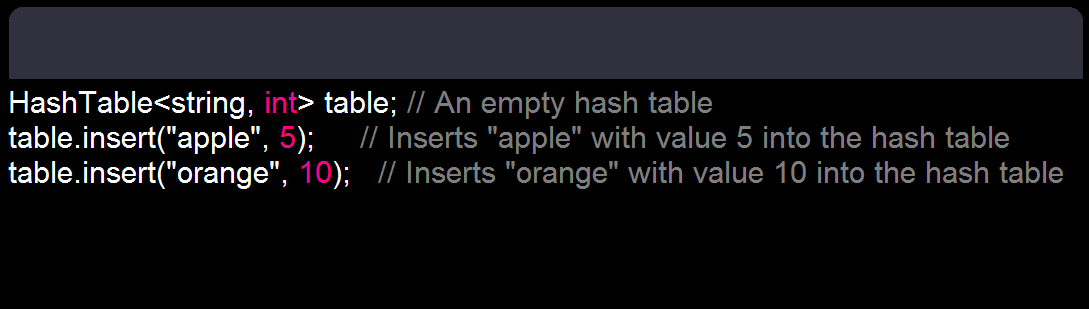
V. Advantages of Variable-Size Data Structures:
- Flexibility: Variable-size data structures adapt to changing data requirements, making them suitable for scenarios where the size of the data collection is unpredictable.
- Memory Efficiency: These data structures allocate memory dynamically, avoiding excessive memory wastage when the collection size is small.
- Reduced Overhead: Variable-size data structures allow for efficient memory utilization, resulting in lower overhead in terms of memory management.
- Optimized Performance: Resizing and memory management are typically handled efficiently by these data structures, ensuring optimal performance during operations.
VI. Use Cases and Applications:
- Handling User Input: Variable-size data structures are well-suited for scenarios where the number of user inputs is not fixed, such as accepting an arbitrary number of elements from a user.
- Dynamic Data Management: When dealing with data collections that grow or shrink over time, variable-size data structures offer an elegant solution.
- File and Database Management: In applications dealing with file data or database records, variable-size data structures can efficiently manage varying amounts of information.
- Data Processing: When processing large datasets with unknown sizes, dynamic data structures help optimize memory utilization.
VII. Considerations:
- Memory Management: While dynamic data structures offer flexibility, they also require careful memory management to avoid memory leaks and fragmentation.
- Resizing Overhead: Dynamic resizing operations may introduce overhead during the resizing process, impacting performance for large data collections.
Conclusion
In conclusion, variable-size data structures are powerful tools in programming, providing flexibility in managing data collections of varying sizes. Dynamic arrays, linked lists, trees, and hash tables are examples of such data structures, each serving specific purposes and applications. The ability to adjust the size of data structures dynamically enhances memory efficiency and allows programmers to handle varying data requirements effectively. When implemented and used correctly, variable-size data structures contribute to efficient and scalable software solutions.
more related content on Principles of Programming Languages