Type checking and type conversions are essential concepts in programming languages, ensuring that operations are performed with compatible data types and facilitating the smooth execution of programs. They play a vital role in maintaining program correctness, avoiding data inconsistencies, and providing flexibility in data representation and manipulation.
Type Checking:
Type checking is the process of verifying the compatibility of data types in a program, ensuring that operations are performed on data of the appropriate types. It is typically performed during compilation in statically-typed languages or at runtime in dynamically-typed languages.
In statically-typed languages like C, C++, and Java, the data types of variables are explicitly defined during declaration. The compiler performs type checking during the compilation phase to detect type errors, such as assigning incompatible data types or using incompatible operations.
Example
in C:

In dynamically-typed languages like Python and JavaScript, variables can change their data type during runtime. Type checking occurs at runtime, and errors related to type incompatibility are identified when the program is executed.
Example
in Python:

Type Conversions (Type Casting):
Type conversion, also known as type casting, is the process of converting data from one data type to another. It allows developers to perform operations between different data types or to store data of one type into a variable of a different type.
Implicit Type Conversion (Coercion):
Implicit type conversion occurs automatically by the programming language when performing operations involving different data types. In this case, the language converts one of the data types to match the other to avoid type errors.
Example in Python:
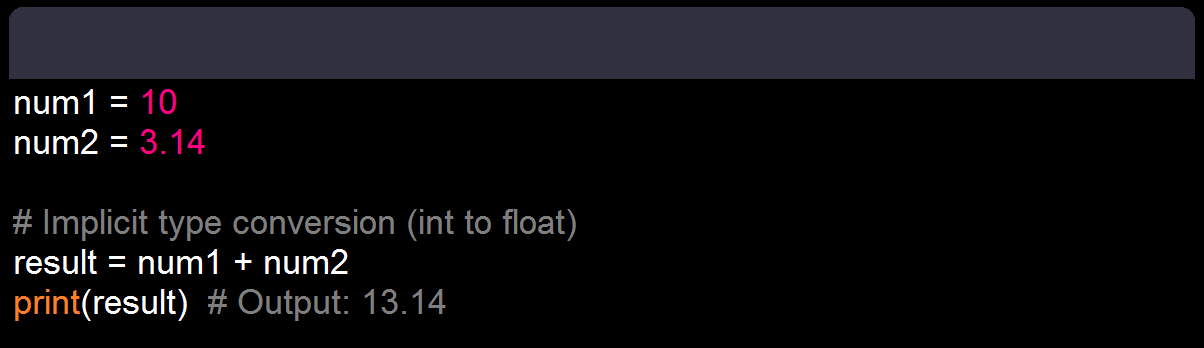
Explicit Type Conversion:
Explicit type conversion is when the developer explicitly converts the data type of a variable using predefined functions or syntax provided by the language.
Example in C:
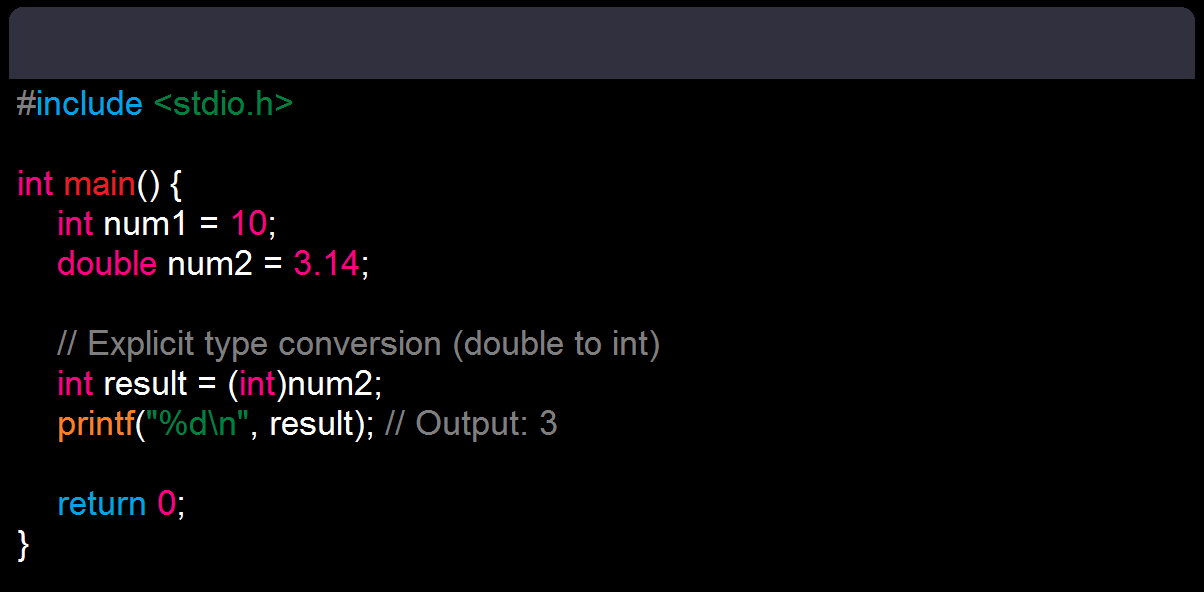
In Python, explicit type conversion is achieved using built-in functions like int(), float(), str(), and others.
Type Checking and Type Conversions in High-Level Languages:
In high-level languages like Python and JavaScript, the approach to type checking and type conversions is more flexible compared to statically-typed languages like C and Java. These languages allow variables to change their data type dynamically, and type checking is done at runtime. While this flexibility simplifies coding and provides more freedom to developers, it also requires careful consideration to avoid unintended type errors and unexpected behavior.
Importance of Type Checking and Type Conversions:
- Program Correctness: Type checking ensures that operations are performed on compatible data types, reducing the risk of runtime errors and improving program correctness.
- Data Consistency: Type conversions allow data to be represented in different formats while ensuring that operations between different data types can be performed without errors.
- Memory Optimization: Type conversions can be used to optimize memory usage by converting data to the most appropriate data type for specific calculations or operations.
- Flexibility: Type conversions enable developers to work with data in different formats, facilitating tasks like input/output processing and data formatting.
- Interoperability: Type conversions are essential when working with external data sources or interacting with other systems that may use different data representations.
Caution with Type Conversions:
While type conversions offer flexibility, developers need to exercise caution to avoid potential data loss or unexpected results. For example, converting from a larger data type to a smaller one may lead to data truncation, resulting in the loss of information.
Example
in C:

In this example, converting the double value 12345.67 to an integer results in the integer variable storing the value 12345. The fractional part is truncated, potentially leading to data loss.
Handling Type Errors:
When handling user input or external data, developers should anticipate potential type errors and implement appropriate error handling mechanisms. Validating and converting user input to the expected data type can prevent runtime errors and improve the user experience.
Summary:
Type checking and type conversions are crucial aspects of programming languages, ensuring data integrity, program correctness, and flexibility in data representation and manipulation. Type checking detects type errors at compile-time or runtime, while type conversions allow for seamless data type transformations. Careful consideration and understanding of type checking and type conversions are essential for writing robust and error-free code in both statically-typed and dynamically-typed programming languages. As developers work with various data types and perform operations between them, a strong grasp of type checking and type conversions significantly improves the efficiency and reliability of their programs.
more related content on Principles of Programming Languages