Sequence Control: Understanding Program Execution Flow
Sequence control is a fundamental concept in computer programming that governs the order in which statements and instructions are executed within a program. It defines the sequence of operations and the flow of control, ensuring that each statement is executed in a predetermined order. This article explores the concept of sequence control, its significance in programming, and its implementation in different programming languages.
Importance of Sequence Control:
In programming, proper sequence control is essential for ensuring the correct execution of a program. The sequence of operations defines the logic of the program and determines the outcome of the computations. Without well-defined sequence control, a program can produce unexpected results and behavior, leading to errors and incorrect outputs.
Basic Sequence Control Structures:
The most basic form of sequence control is a linear sequence, where statements are executed in the order they appear in the code. This is the default behavior in most programming languages.
Example of Linear Sequence in C:
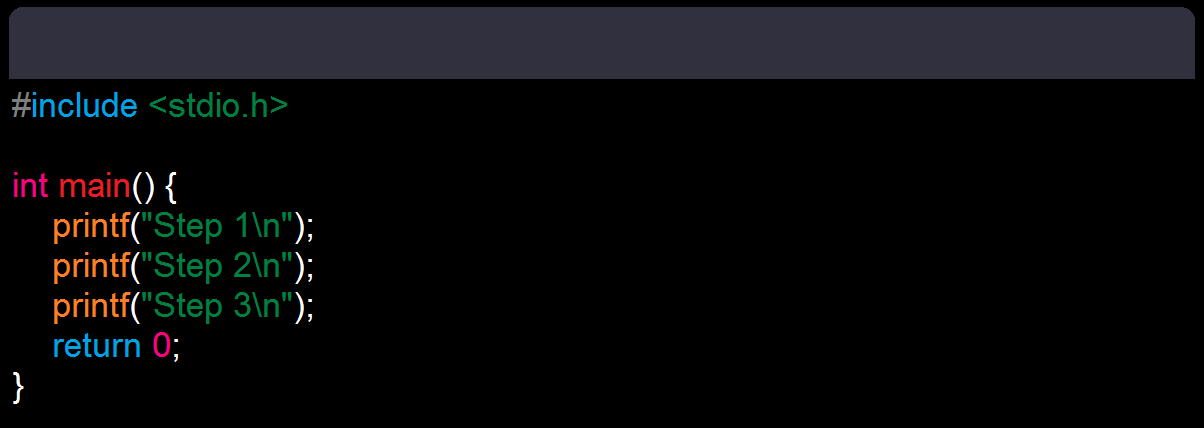
In this C code snippet, the statements are executed in a linear sequence: “Step 1” is printed first, followed by “Step 2” and “Step 3” in order.
Conditional Sequence Control:
Conditional structures, such as if-else statements and switch statements, allow the program to follow different sequences of statements based on certain conditions.
Example of Conditional Sequence Control in Python:
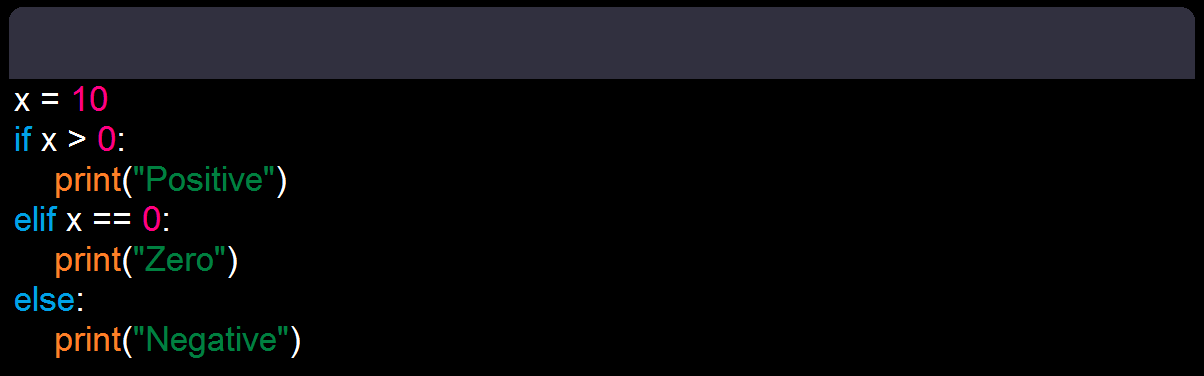
In this Python code snippet, the program uses conditional sequence control to print whether the value of x is positive, zero, or negative.
Looping Sequence Control:
Looping structures, such as for loops and while loops, allow the program to repeat a sequence of statements multiple times.
Example of Looping Sequence Control in Java:

In this Java code snippet, the program uses a for loop to print “Iteration 0” to “Iteration 4” in sequence.
Subroutine Sequence Control:
Subroutines (functions or methods) provide a way to group sequences of statements into reusable blocks of code. By calling subroutines, programmers can execute specific sequences of statements at different points in the program.
Example of Subroutine Sequence Control in C++:
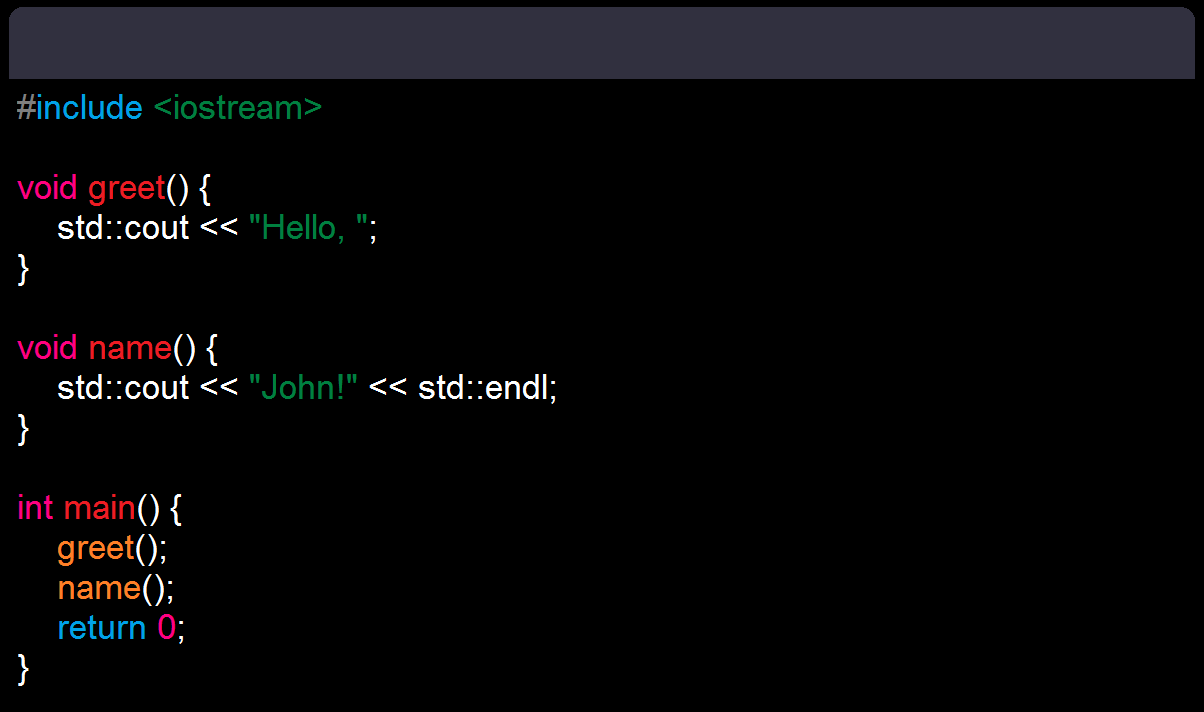
In this C++ code snippet, the program uses two subroutines, greet and name, to print “Hello, ” and “John!” respectively. By calling these subroutines in the main function, the program executes the desired sequence of statements.
Error Handling and Sequence Control:
Error handling is an essential aspect of sequence control. When exceptions or errors occur during program execution, the control flow may need to be redirected to handle the exceptional condition.
Example of Error Handling in Python:
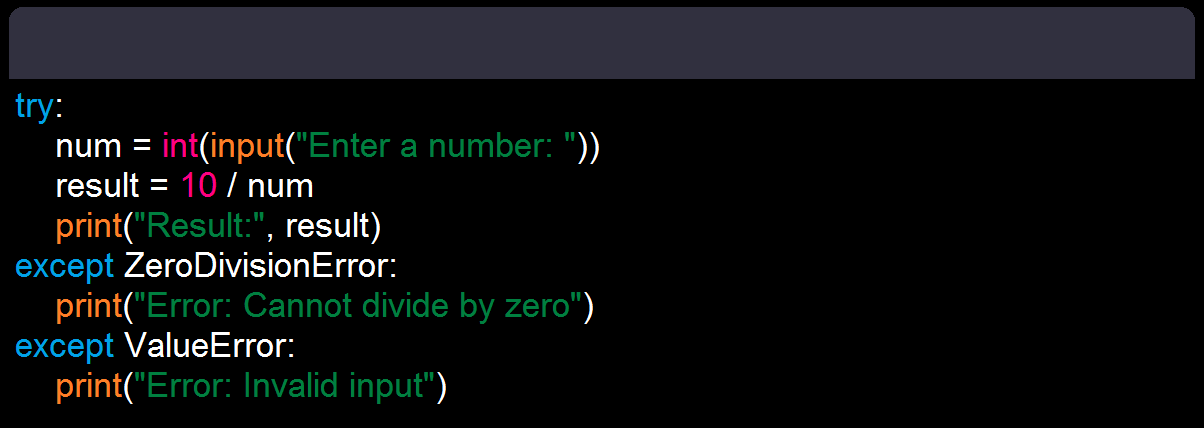
In this Python code snippet, the program handles two types of exceptions: ZeroDivisionError when the user enters zero, and ValueError when the user enters a non-numeric input.
The Role of Sequence Control in Algorithms:
Sequence control is a fundamental aspect of algorithm design. Properly sequencing operations and controlling the flow of execution are crucial for designing efficient algorithms and solving complex problems.
Parallelism and Sequence Control:
In modern programming paradigms, parallelism allows multiple tasks to be executed concurrently. However, parallelism requires careful management of sequence control to avoid race conditions and ensure data consistency.
Conclusion:
Sequence control is the backbone of program execution, defining the order in which statements are executed and the flow of control within a program. By leveraging conditional structures, looping structures, subroutines, and error handling mechanisms, programmers can design robust and efficient programs. Proper sequence control is crucial for producing correct outputs, managing program flow, and ensuring the reliability of software applications. As programmers continue to innovate and explore new programming paradigms, sequence control remains a core concept that underpins the logical and structured design of computer programs.
more related content on Principles of Programming Languages