Sequence Control Within Statements: Controlling Flow in Code Blocks
Sequence control within statements is a critical aspect of programming that governs the order of statement execution within code blocks. Code blocks are groups of statements enclosed within curly braces {} in many programming languages. Managing the flow of execution within these blocks ensures that statements are executed in the desired order, and it directly impacts the logic and behavior of a program.
The Importance of Statement Sequence Control:
In programming, statements are the fundamental building blocks that perform actions or calculations. The order in which these statements are executed determines the overall functionality and outcome of a program. Sequence control within statements allows developers to structure their code effectively, handle conditional operations, and perform repetitive tasks.
Implicit Sequence Control:
By default, statements within a code block are executed sequentially, following the order in which they are written. This implicit sequence control is the simplest form of flow, where statements are executed one after the other.
Example of Implicit Sequence Control:

In this Python code snippet, the statements are executed in the order they appear. First, the variable x is assigned the value 5, then y is assigned the value 10, and finally, the sum of x and y is assigned to z, which is then printed. The sequence control is implicit, as there are no control flow statements like conditionals or loops that alter the natural order of execution.
Conditional Statements:
Conditional statements, such as if, else if, and else, are powerful tools for sequence control within statements. They allow the program to make decisions based on specific conditions and execute different blocks of code accordingly.
Example of Conditional Sequence Control:
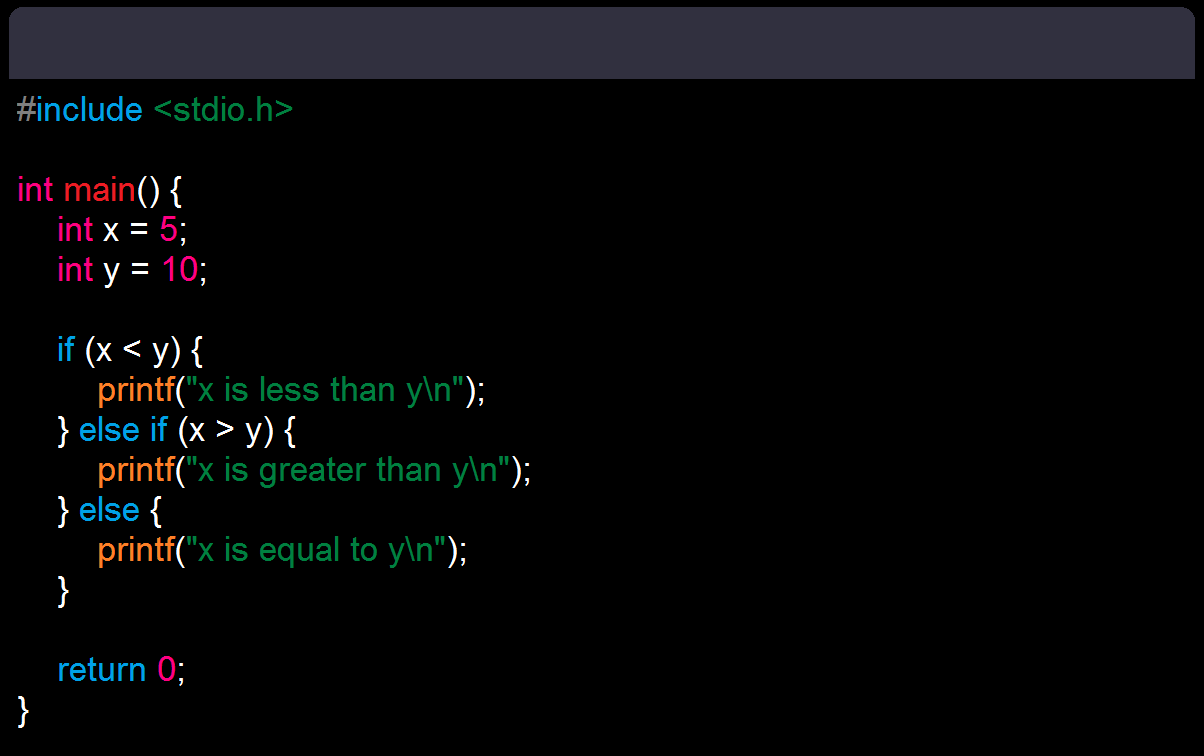
In this C code snippet, the if, else if, and else statements are used for conditional sequence control. Depending on the values of x and y, the program executes different branches of code to print the appropriate message.
Loops:
Loops are another form of sequence control that allow repetitive execution of a block of code until a specific condition is met. They enable developers to perform actions multiple times without writing redundant code.
Example of Loop Sequence Control:

In this Python code snippet, the for loop is used for loop sequence control. The loop iterates five times, and the print statement is executed with different values of i in each iteration.
Jump Statements:
Jump statements, such as break, continue, and return, provide additional control over the sequence of statements within a code block. They allow developers to alter the flow of execution or exit the loop or function prematurely.
Example of Jump Sequence Control:

In this C code snippet, the break statement is used to exit the for loop when the value of i is 5. As a result, the loop terminates prematurely when the condition is met.
Nested Sequence Control:
In more complex scenarios, developers may use nested sequence control, combining conditional statements, loops, and jump statements to create intricate program logic.
Example of Nested Sequence Control:

In this Python code snippet, the for loop is combined with an if statement and the continue statement. The loop iterates five times, and when i is even, the continue statement skips the remaining code in that iteration and moves on to the next value of i.
Importance of Proper Sequence Control:
Correct sequence control is essential to ensure that statements are executed in the desired order, especially in complex programs with multiple conditions and loops. Proper sequence control enhances code readability, maintainability, and debugging efficiency.
Avoiding Infinite Loops:
Careful sequence control is crucial to avoid infinite loops, where a loop continues indefinitely without a proper exit condition. Infinite loops can cause programs to hang or crash and are a common source of bugs.
Example of Infinite Loop:
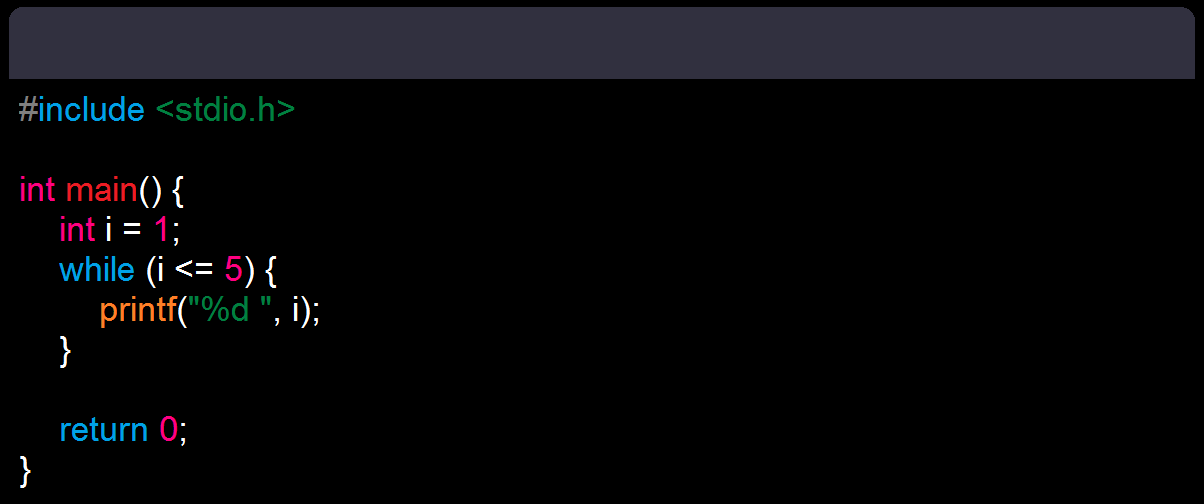
In this C code snippet, the loop never reaches an exit condition, and the program enters an infinite loop, continuously printing the value of i.
Conclusion:
Sequence control within statements is a fundamental aspect of programming that determines the order of statement execution within code blocks. Implicit sequence control follows the natural order of statements, while explicit sequence control uses conditional statements, loops, and jump statements to modify the flow of execution. Proper sequence control is vital to creating well-structured and efficient programs, enhancing readability, maintainability, and debugging capabilities. By mastering sequence control, programmers can design complex algorithms and logic that handle diverse real-world scenarios effectively. Understanding the nuances of sequence control is an essential skill for any proficient developer seeking to build reliable and robust software solutions.
more related content on Principles of Programming Languages