Sequence Control Within Expressions: Managing Order of Evaluation in Complex Expressions
In programming, expressions are combinations of variables, constants, and operators that are evaluated to produce a single value. When dealing with complex expressions, the order in which the subexpressions are evaluated can significantly impact the final result. Sequence control within expressions refers to the rules and mechanisms that determine the order of evaluation of subexpressions in a larger expression. Proper management of sequence control is crucial to ensure accurate and predictable results in programming languages.
Implicit Sequence Control in Expressions:
In most programming languages, expressions are evaluated implicitly using a set of predefined rules, also known as the default evaluation order. These rules dictate the order in which subexpressions within a larger expression are evaluated. The most common rule is to follow the precedence and associativity of operators to determine the sequence of evaluation.
Example of Implicit Sequence Control in Expressions:
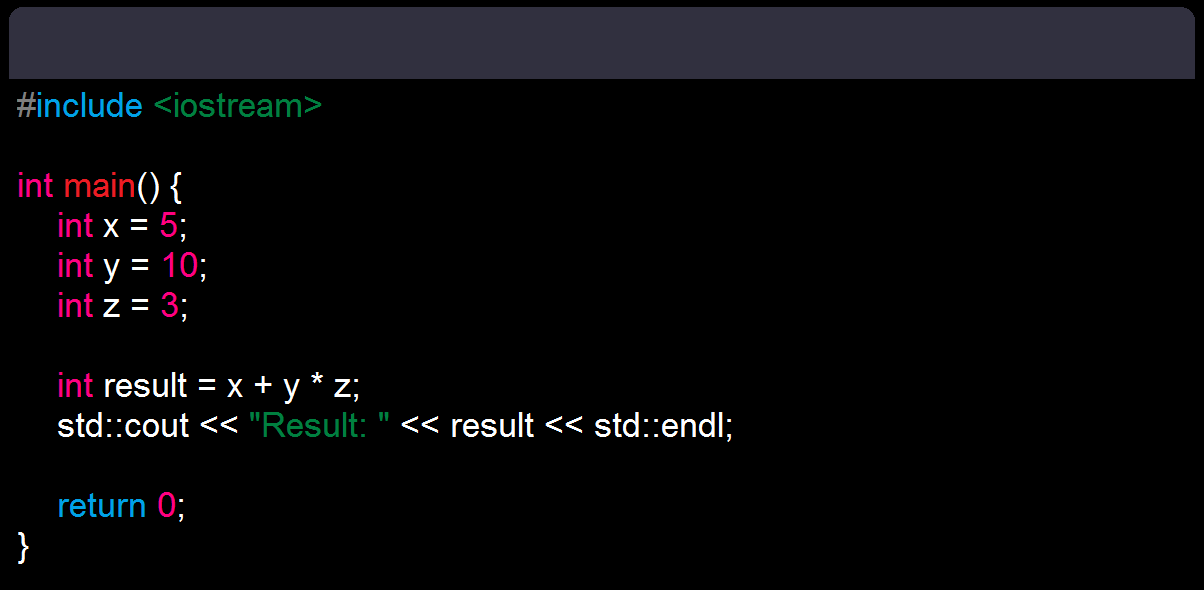
In this C++ code snippet, the expression x + y * z contains two operators: + and *. According to the default evaluation order, the multiplication operation y * z is performed before the addition x + (y * z). This is because the multiplication operator has higher precedence than the addition operator.
Operator Precedence and Associativity:
Operator precedence defines the priority of operators, determining which operation should be performed first. For example, multiplication and division have higher precedence than addition and subtraction. Associativity, on the other hand, defines the order in which operations of equal precedence are performed. Operators can be left-associative (evaluated from left to right) or right-associative (evaluated from right to left).
Example of Operator Precedence and Associativity:
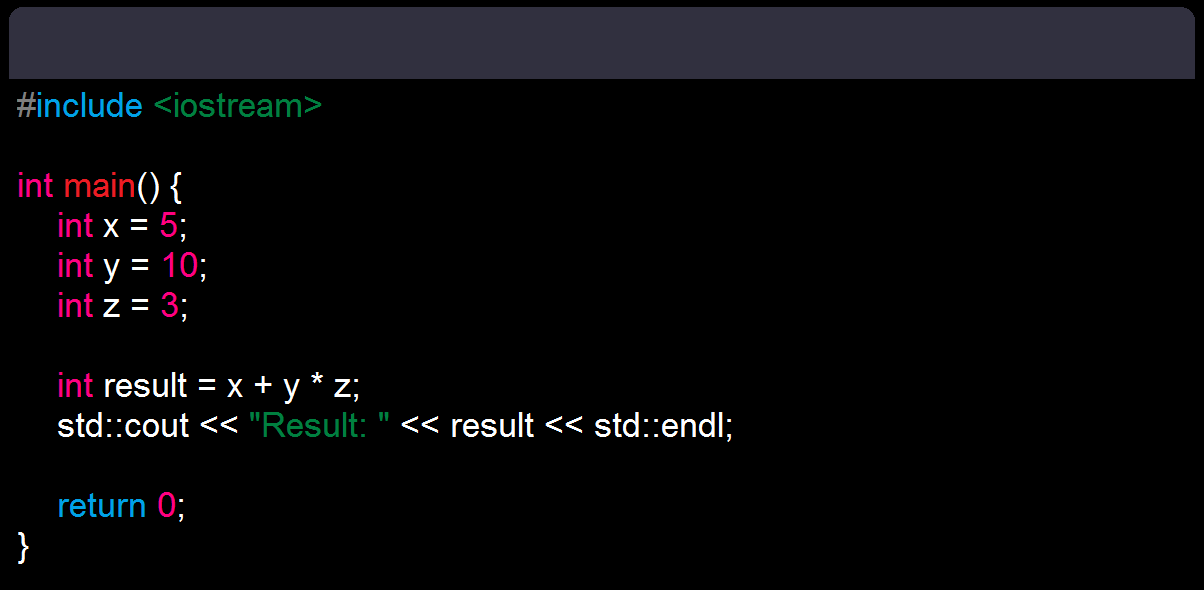
In this C++ code snippet, the expression x + y * z follows the operator precedence and associativity rules. The multiplication y * z is performed first due to higher precedence, and then the addition x + (y * z) is evaluated.
Side Effects and Order of Evaluation:
While the default evaluation order ensures consistent results, it is essential to be aware of side effects within expressions. Side effects occur when an expression modifies a variable or has an observable effect beyond its value. The order of evaluation can impact the outcome when side effects are present.
Example of Side Effects and Order of Evaluation:

In this C++ code snippet, the expression x + y++ * z contains the post-increment operator y++. The order of evaluation can affect the final value of y and, consequently, the result of the expression. If the multiplication is evaluated before the increment, the result is x + (y * z), but if the increment is performed first, the result becomes x + ((y + 1) * z).
Explicit Sequence Control:
Parentheses and Evaluation Order: To enforce a specific order of evaluation in complex expressions, explicit sequence control can be achieved using parentheses. By enclosing subexpressions within parentheses, developers can ensure that those subexpressions are evaluated first, regardless of the default evaluation order.
Example of Explicit Sequence Control with Parentheses:

In this C++ code snippet, the expression (x + y) * z uses parentheses to enforce the addition x + y to be evaluated before the multiplication * z.
Conditional and Short-Circuit Evaluation:
In some programming languages, such as C and C++, expressions involving logical operators (&& and ||) may utilize conditional evaluation or short-circuit evaluation. In short-circuit evaluation, if the result of an expression can be determined based on the left operand alone, the right operand is not evaluated.
Example of Short-Circuit Evaluation:
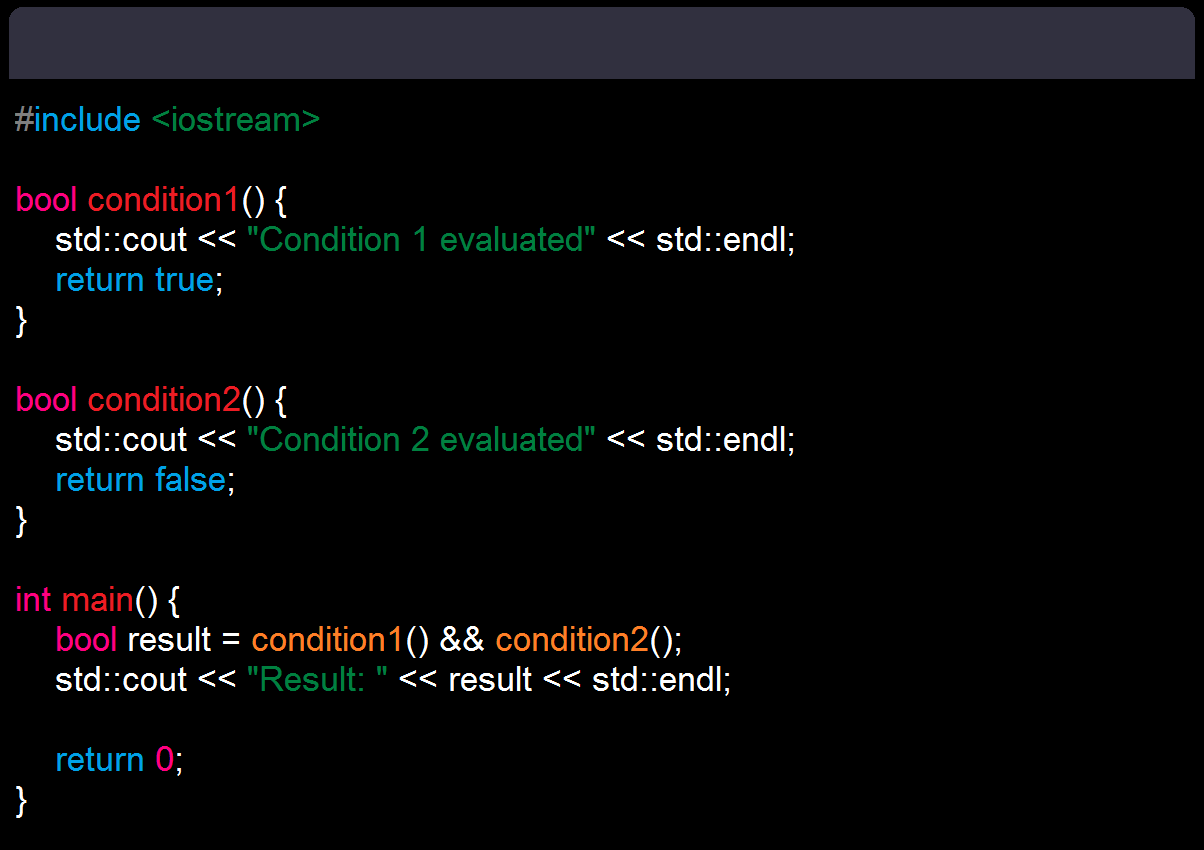
In this C++ code snippet, the expression condition1() && condition2() involves the logical AND operator &&. Since the left operand (condition1()) evaluates to true, the right operand (condition2()) is not evaluated, as the result of the expression is already determined (short-circuit evaluation).
Conclusion:
Sequence control within expressions is a crucial aspect of programming that governs the order of evaluation in complex expressions. Implicit sequence control, based on operator precedence and associativity, is the default approach used by most programming languages. Understanding the default evaluation order is essential for producing accurate results. However, explicit sequence control using parentheses can be employed to enforce a specific order of evaluation. Additionally, developers should be mindful of side effects within expressions and consider the use of short-circuit evaluation for logical expressions. Proper management of sequence control is vital for writing correct, efficient, and reliable code that meets the requirements of diverse real-world scenarios.
more related content on Principles of Programming Languages