Recursive Subprograms: Exploring the Power of Self-Reference
In computer programming, a recursive subprogram is a function or procedure that calls itself during its execution. This powerful technique allows programmers to solve complex problems by breaking them down into smaller, more manageable subproblems. Recursive subprograms are widely used in various programming languages and play a crucial role in solving problems involving repetitive patterns or hierarchical structures. This article explores the concept of recursive subprograms, their benefits, working principles, and common use cases.
Understanding Recursive Subprograms:
Recursive subprograms are based on the concept of self-reference, where a function or procedure invokes itself within its definition. This self-referential property allows the subprogram to perform repetitive tasks or solve problems by reducing them to smaller, identical subproblems. The key element in a recursive subprogram is the “base case,” which defines when the recursion should stop and the function should return a result.
Recursive Function Example: Factorial Calculation:
One classic example of a recursive subprogram is the calculation of factorial, denoted as n!. The factorial of a non-negative integer n is the product of all positive integers less than or equal to n. The base case for factorial is 0! = 1, as the factorial of 0 is defined as 1.
Example of Recursive Function to Calculate Factorial in C++:
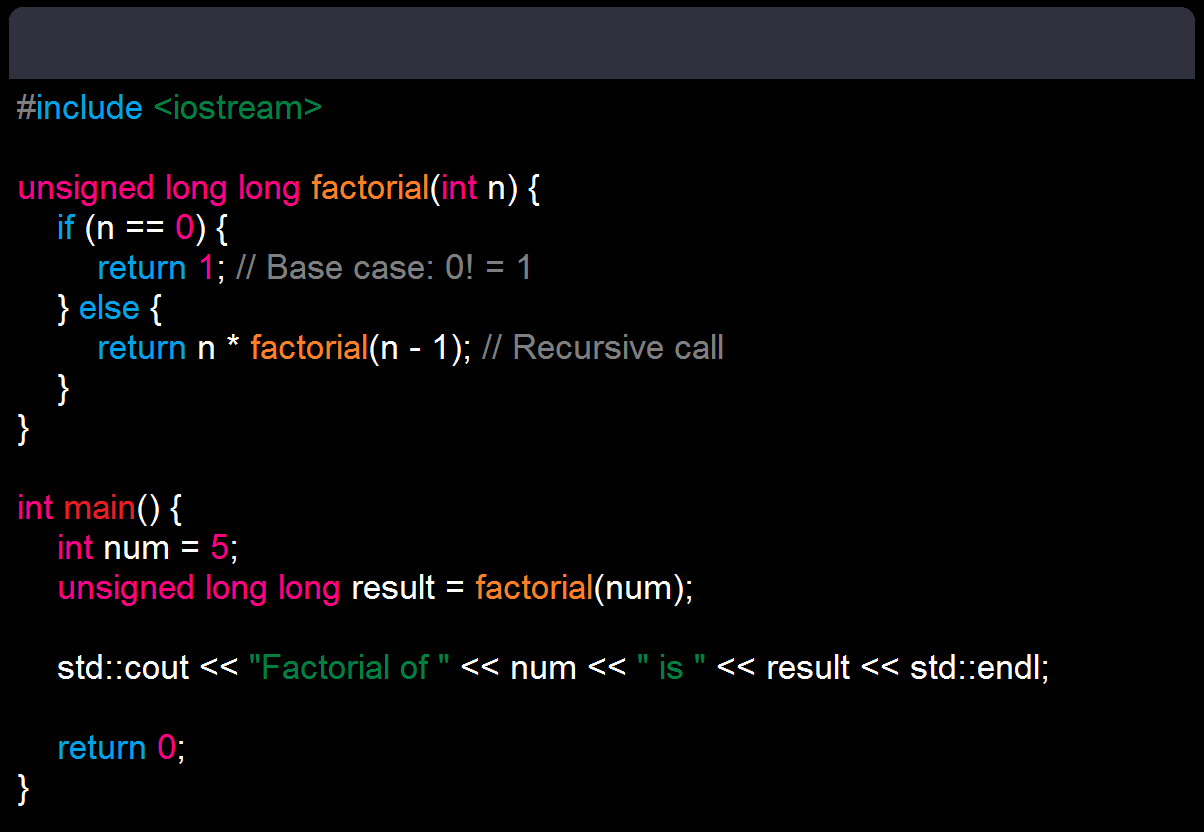
In this C++ code snippet, the factorial function is a recursive subprogram that calculates the factorial of a given non-negative integer n. It calls itself with a smaller value of n until it reaches the base case (when n is 0), and then returns the final result.
How Recursive Subprograms Work:
When a recursive subprogram is called, it creates a new instance of the function on the call stack, known as a “recursive stack frame.” Each stack frame has its own set of parameters, local variables, and return address. As the recursion progresses, multiple stack frames are created and organized in a last-in, first-out (LIFO) manner.
The Importance of Base Case:
The base case is a critical aspect of recursive subprograms. It defines the stopping condition for the recursion and prevents an infinite loop. Without a base case, the recursive subprogram would call itself indefinitely, leading to a stack overflow and program crash.
Common Use Cases of Recursive Subprograms:
- Mathematical Operations: Recursive subprograms are used to compute mathematical functions like Fibonacci numbers, factorials, and exponentiation efficiently.
- Data Structures: Many data structures, such as linked lists, trees, and graphs, can be easily traversed or searched using recursive subprograms.
- Sorting and Searching Algorithms: Recursive techniques are employed in sorting algorithms like Merge Sort and Quick Sort.
- Parsing and Syntax Analysis: Recursive descent parsing is a common method used in language processing to recognize grammar and construct abstract syntax trees.
- Backtracking: Recursive subprograms are used in backtracking algorithms to solve problems with multiple potential solutions.
Pros and Cons of Recursive Subprograms:
Pros:
- Elegant Code: Recursive solutions often lead to more concise and elegant code, making the implementation easier to understand.
- Simplification of Complex Problems: Recursive subprograms help in breaking down complex problems into smaller, manageable subproblems, simplifying the overall solution.
- Code Reusability: Recursive functions can be reused to solve similar problems with different inputs.
Cons:
- Performance Overhead: Recursive subprograms may lead to a higher overhead due to the creation of multiple stack frames.
- Stack Overflow Risk: If not properly managed, excessive recursion can lead to a stack overflow error.
Tips for Using Recursive Subprograms:
- Define the Base Case Carefully: Ensure the base case is correctly defined to prevent infinite recursion.
- Optimize Tail Recursion: Tail recursion optimization is a compiler feature that converts tail-recursive calls into iterative loops, reducing the stack space required.
- Use Iterative Solutions Where Appropriate: While recursion is powerful, it may not always be the most efficient solution. Consider using iterative approaches for certain problems.
Conclusion:
Recursive subprograms are a powerful tool in a programmer’s toolkit, offering an elegant and effective way to solve complex problems through self-reference. By understanding how recursive functions work, mastering the base case concept, and implementing appropriate termination conditions, programmers can leverage recursive subprograms to develop efficient and elegant solutions to a wide range of problems. While recursion may introduce performance overhead, careful use of this technique can lead to code that is both easier to understand and maintain. As a fundamental concept in computer science, recursive subprograms continue to play a significant role in programming and algorithm design, facilitating the development of innovative and sophisticated software solutions.
more related content on Principles of Programming Languages