Introduction
Priority Queue is a crucial data structure that plays a vital role in computer science and programming. It is widely used in various algorithms and applications, including operating systems, network routing, data compression, and more. Priority Queue is a type of queue that assigns a priority to each element and allows them to be retrieved in a specific order based on their priority.
What is Priority Queue in DAA?
Priority Queue is a special type of queue that assigns a priority to each element and allows the elements to be retrieved in a specific order based on their priority. Priority Queue in DAA is used to implement various algorithms that require elements to be processed in a specific order.
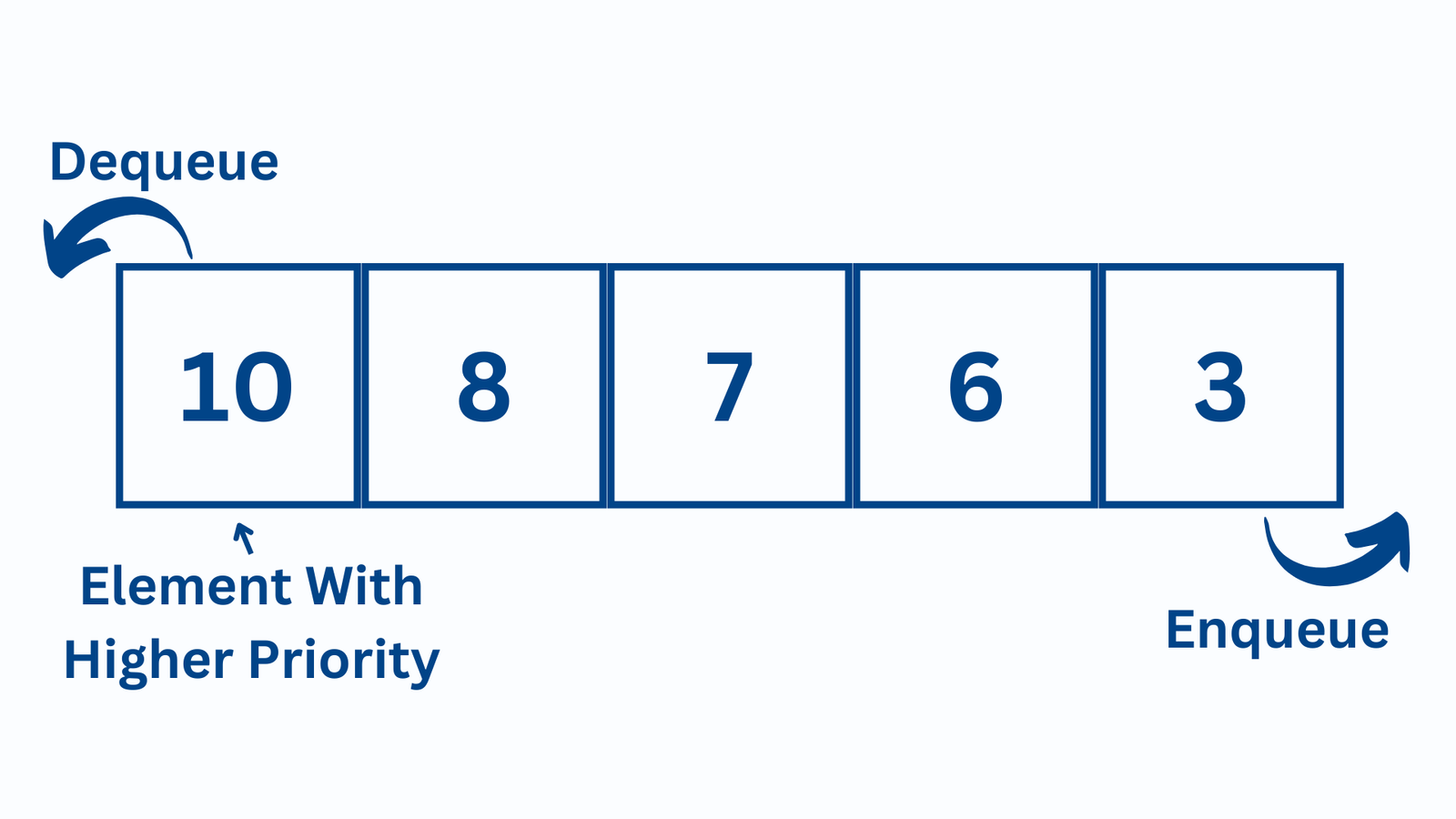
Priority Queue in DAA can be visualized as a binary heap, which is a complete binary tree where the value of each parent node is greater than or equal to the values of its children nodes. The root node of the binary heap is the element with the highest priority, and the leaf nodes are the elements with the lowest priority.
Types of Priority Queue
There are two types of Priority Queue:
1. Min-Heap
A Min-Heap is a Priority Queue where the element with the lowest priority has the highest value. In a Min-Heap, the root node has the minimum value, and each parent node has a lower value than its children nodes.
2. Max-Heap
A Max-Heap is a Priority Queue where the element with the highest priority has the highest value. In a Max-Heap, the root node has the maximum value, and each parent node has a higher value than its children nodes.
Operations on Priority Queue
Priority Queue supports the following operations:
1. Insert
The Insert operation is used to add an element to the Priority Queue. The element is inserted in such a way that the Priority Queue property is maintained.
2. Delete
The Delete operation is used to remove the element with the highest priority from the Priority Queue. The highest priority element is always at the root of the Priority Queue.
3. Peek
The Peek operation is used to retrieve the element with the highest priority from the Priority Queue without removing it.
Priority Queue Code in C++
#include <iostream> #include <queue> using namespace std; int main() { // Declaring a priority queue of integers priority_queue<int> pq; // Pushing elements into the priority queue pq.push(3); pq.push(2); pq.push(5); pq.push(1); pq.push(4); // Printing the top element of the priority queue cout << "Top element: " << pq.top() << endl; // Removing the top element of the priority queue pq.pop(); // Printing the top element of the priority queue after removal cout << "Top element after removal: " << pq.top() << endl; // Checking if the priority queue is empty if (pq.empty()) { cout << "Priority queue is empty" << endl; } else { cout << "Priority queue is not empty" << endl; } // Printing the size of the priority queue cout << "Size of priority queue: " << pq.size() << endl; // Adding more elements to the priority queue pq.push(7); pq.push(6); // Printing the top element of the priority queue cout << "Top element: " << pq.top() << endl; // Removing all elements from the priority queue while (!pq.empty()) { pq.pop(); } // Printing the size of the priority queue after removal of all elements cout << "Size of priority queue after removal of all elements: " << pq.size() << endl; return 0; }
Applications of Priority Queue
Priority Queue has a wide range of applications in computer science and programming. Some of the common applications of Priority Queue are:
1. Dijkstra’s Algorithm
Dijkstra’s Algorithm is a shortest path algorithm that uses Priority Queue to find the shortest path between two nodes in a graph.
2. Huffman Coding
Huffman Coding is a data compression algorithm that uses Priority Queue to generate a variable-length prefix code for each symbol in a given data stream.
3. Operating Systems
Priority Queue is used in operating systems to implement various scheduling algorithms, such as Round Robin, Priority Scheduling, and Shortest Job First.
4. Network Routing
Priority Queue is used in network routing algorithms to route packets based on their priority.
Advantages of Priority Queue
- It allows us to process the elements in order of priority, which can be more
- It provides a simple and efficient way to implement certain algorithms and data structures, such as Dijkstra’s algorithm and Huffman coding.
- It allows us to efficiently manage resources in a system by assigning priorities to them and processing them accordingly.
Disadvantages of Priority Queue
- Priority queues can be more complex to implement and use than regular queues, especially for beginners.
- Depending on the implementation, some operations on a priority queue can be slower than on a regular queue.
- It can be challenging to balance the priorities of different elements, especially if they have similar priority levels.
Priority Queue vs. Regular Queue
The main difference between a priority queue and a regular queue is the order in which the elements are processed. In a regular queue, the elements are processed in the order in which they were added to the queue, whereas in a priority queue, the elements are processed based on their priority levels.
FAQs
- What is the difference between a priority queue and a regular queue?
A priority queue processes elements based on their priority levels, whereas a regular queue processes elements in the order in which they were added.
- What is the most common implementation of a priority queue?
The most common implementation of a priority queue is using a heap data structure.
- What are some applications of a priority queue?
Priority queues are used in Dijkstra’s algorithm, Huffman coding, operating system scheduling, and more.
- Can a priority queue have elements with the same priority level?
Yes, a priority queue can have elements with the same priority level, and their order is determined by the order in which they were added.
- Why is a priority queue important?
A priority queue is important because it allows us to efficiently manage our data by prioritizing the elements based on their importance or urgency.