Pointers are a fundamental concept in programming that allows manipulation and direct access to memory addresses. They are used to store the memory addresses of variables or data structures, enabling efficient memory management and direct memory access. Pointers play a crucial role in low-level programming and are essential for tasks like dynamic memory allocation, data structures implementation, and interacting with hardware devices.
Pointer Declaration and Initialization:
In most programming languages that support pointers, the declaration and initialization of a pointer involve using an asterisk (*) symbol before the variable name to indicate that it is a pointer. Pointers are typically initialized with the address of another variable, using the ampersand (&) operator to retrieve the memory address.
Example
in C:
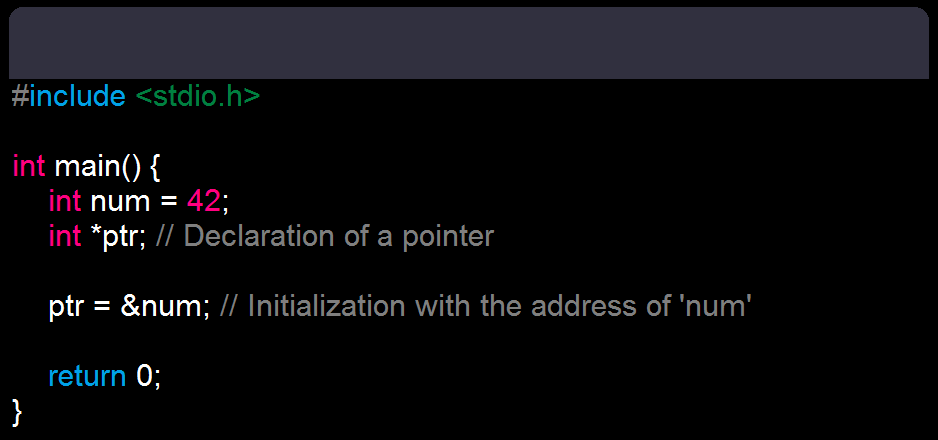
Dereferencing Pointers:
Dereferencing a pointer means accessing the value stored at the memory address pointed to by the pointer. It is achieved by using the asterisk (*) symbol before the pointer variable name. Dereferencing allows manipulation of the value at the memory address directly.
Example
in C:
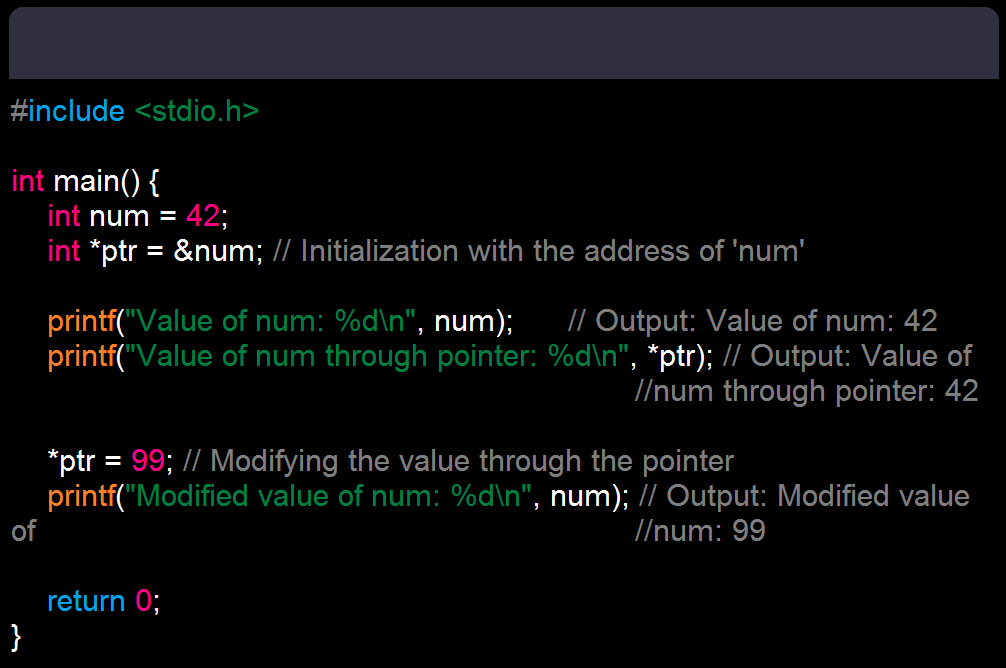
Pointers and Dynamic Memory Allocation:
One of the primary applications of pointers is dynamic memory allocation, which allows programs to allocate memory at runtime. This is particularly useful when the size of data structures is not known at compile-time or when dealing with large data sets.
Example
in C:
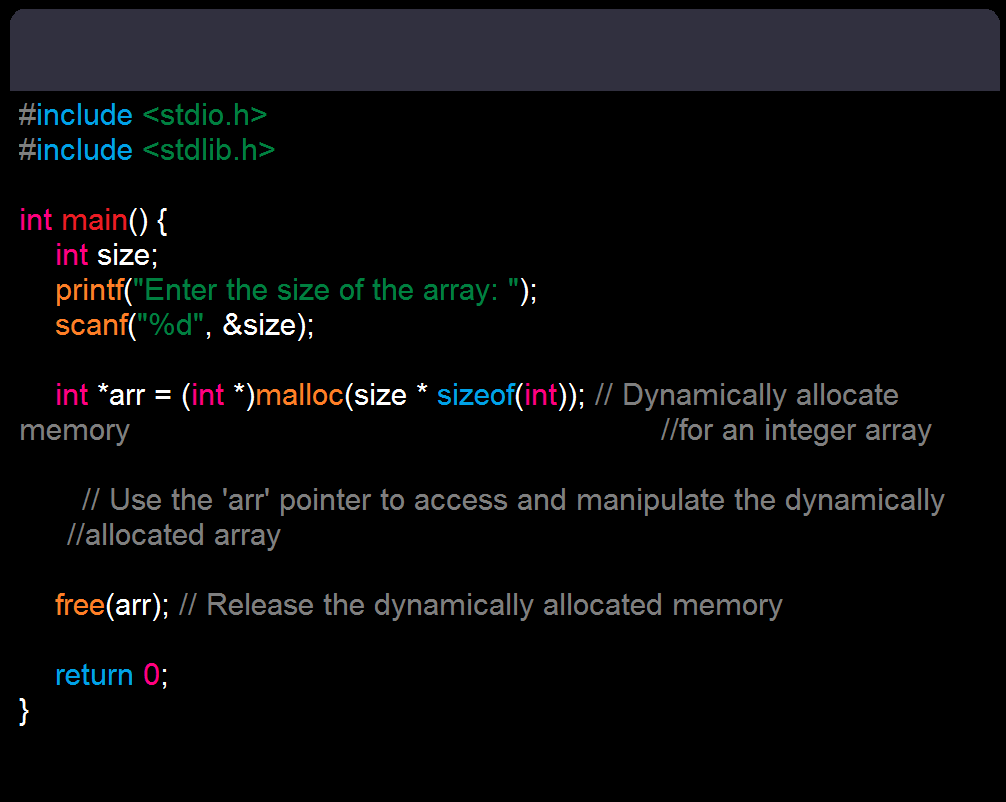
Pointers and Data Structures:
Pointers are extensively used in data structures implementation, as they provide efficient ways to traverse and manipulate linked data structures like linked lists, trees, and graphs. By pointing to the memory address of the next node or element, pointers allow seamless navigation through data structures.
Example
in C:
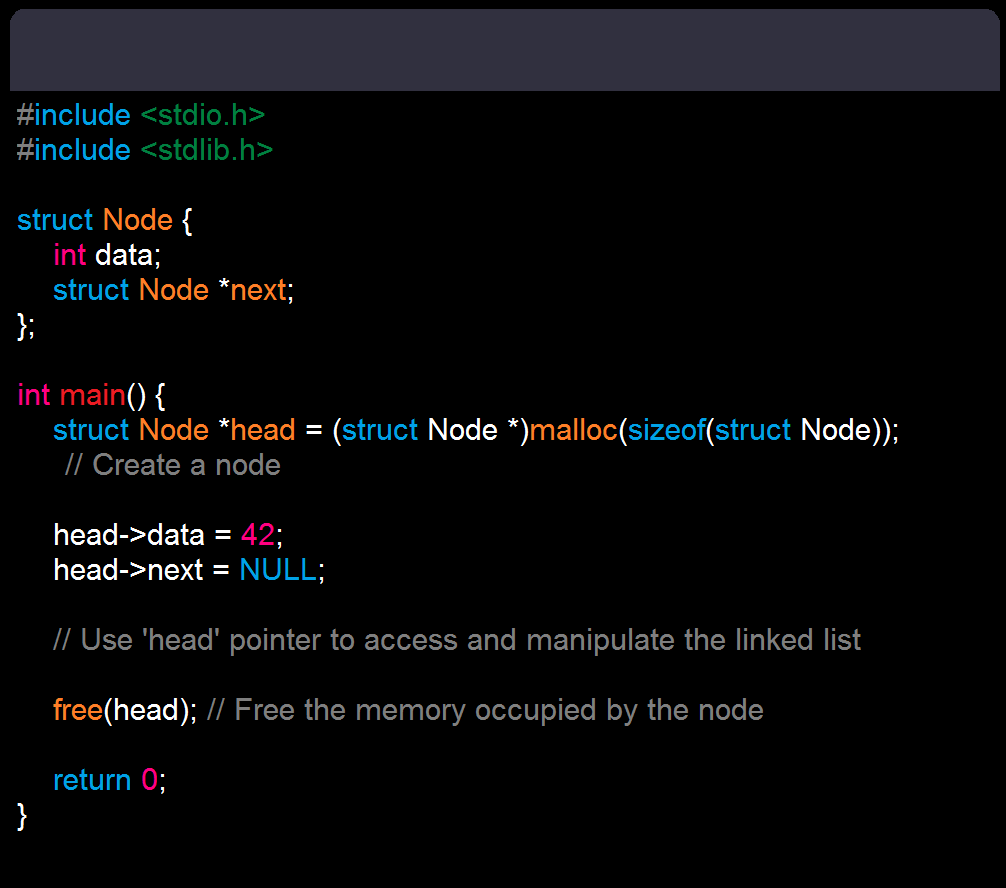
Pointers and Function Parameters:
Pointers are often used in functions to pass variables by reference instead of by value. By passing the address of a variable as a pointer, a function can directly modify the original variable in memory.
Example
in C:
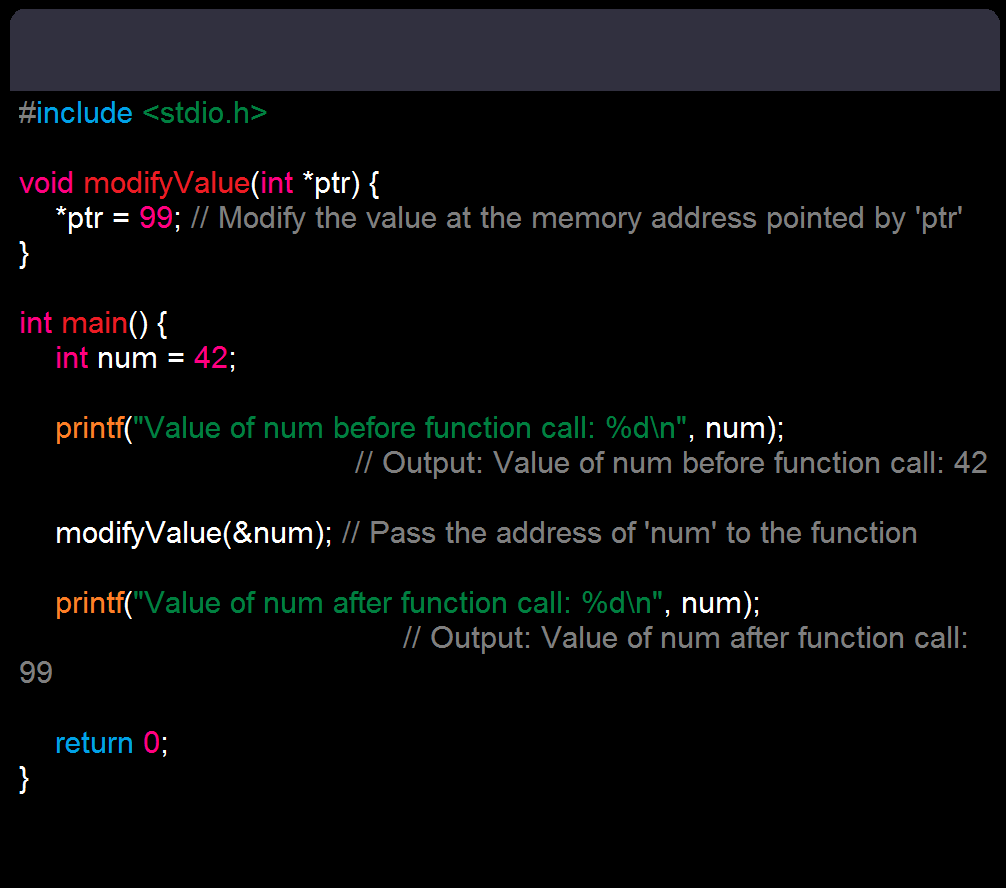
Pointers and Arrays:
In many programming languages, arrays are internally represented as pointers to the first element of the array. This means that the name of the array itself acts as a pointer to its first element, enabling efficient access to array elements using pointer arithmetic.
Example
in C:
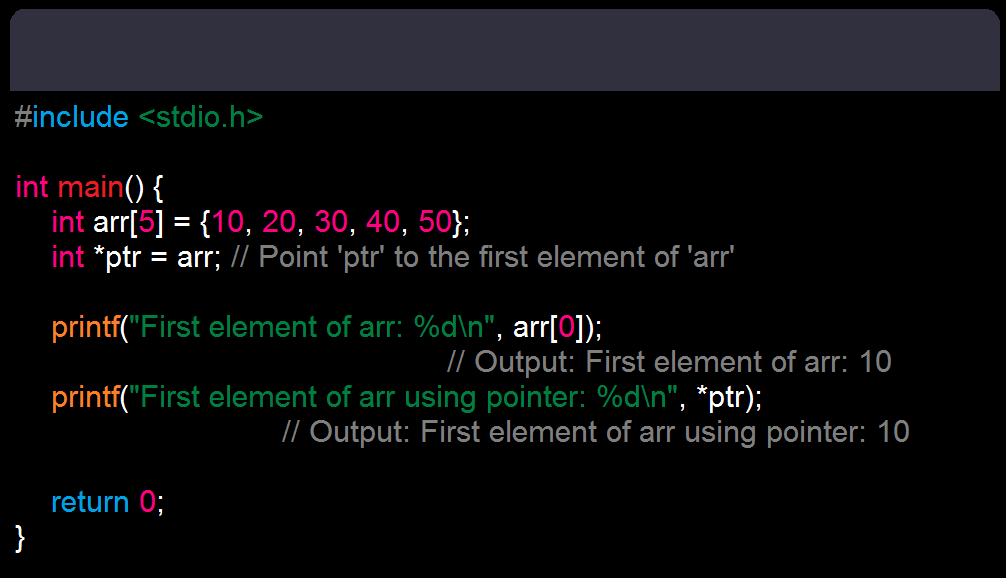
Null Pointers:
A null pointer is a special pointer value that points to no memory location. It is used to indicate that a pointer does not currently point to a valid memory address. In languages like C and C++, null pointers are commonly used to initialize pointers before allocating memory or when a pointer is no longer needed.
Example
in C:
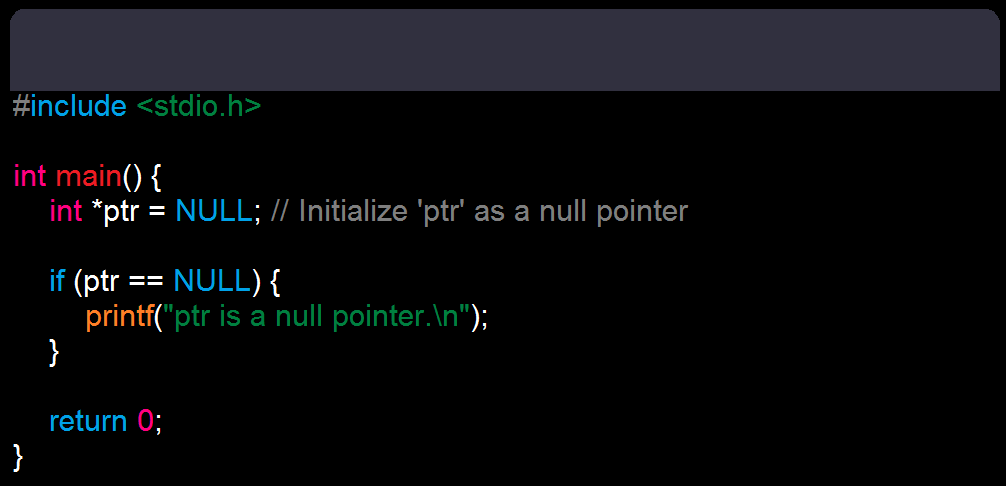
Pointers and Type Safety:
Using pointers requires careful handling to ensure type safety and prevent unintended memory access errors. Dereferencing an uninitialized or invalid pointer can lead to undefined behavior and program crashes. It is essential to initialize pointers correctly and ensure they point to valid memory locations before dereferencing them.
Memory Management and Pitfalls:
While pointers offer flexibility and memory management capabilities, they also come with certain pitfalls, such as memory leaks and dangling pointers. Memory leaks occur when dynamically allocated memory is not deallocated properly, leading to memory wastage. Dangling pointers arise when a pointer points to memory that has already been deallocated, resulting in undefined behavior when accessed.
Pointers in High-Level Programming:
While pointers are crucial in low-level programming for tasks like memory management and hardware interaction, high-level languages often abstract pointers away from developers to improve safety and simplify coding. High-level languages often manage memory automatically through garbage collection or smart pointers, reducing the risk of memory-related errors.
Conclusion
In conclusion, pointers are a powerful and essential concept in programming, providing direct access to memory addresses and enabling efficient memory management. They play a crucial role in low-level programming, data structures implementation, and dynamic memory allocation. However, they require careful handling to ensure type safety and avoid pitfalls like memory leaks and dangling pointers. As developers progress to high-level languages, pointers are often abstracted away to simplify coding and improve memory management through automatic memory management mechanisms. Understanding pointers is vital for mastering low-level programming and gaining a deeper understanding of memory management in software development.
more related content on Principles of Programming Languages