Table of contents
Introduction
Have you ever faced difficulties in managing data in your programs? Let’s say you have a large number of data items that you need to store and retrieve frequently. How would you do that? You could use an array, but what if the number of items is constantly changing? In such cases, linked lists come to the rescue.
What is Linked Lists ?
Linked lists are a type of data structure in which data items are stored in nodes, and these nodes are linked together. Each node contains a data item and a pointer to the next node in the list. The first node is called the head, and the last node is called the tail.

Types of Linked Lists
There are three types of linked lists: singly linked lists, doubly linked lists, and circular linked lists.
Singly Linked Lists
In singly linked lists, each node has a pointer to the next node in the list. The last node has a pointer to null.
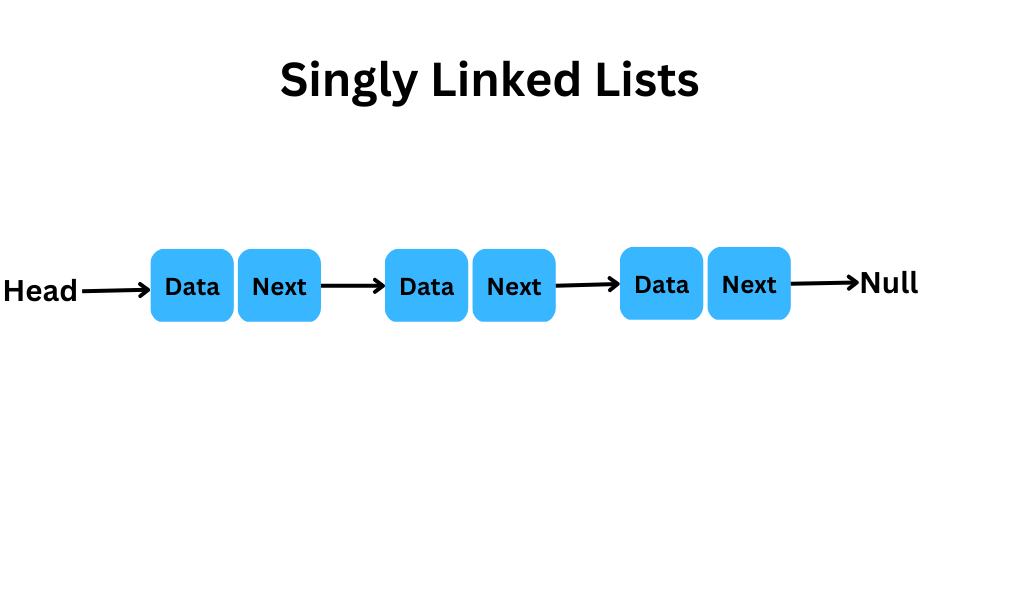
Doubly Linked Lists
In doubly linked lists, each node has a pointer to both the next and the previous nodes. This allows for more efficient insertion and deletion operations.
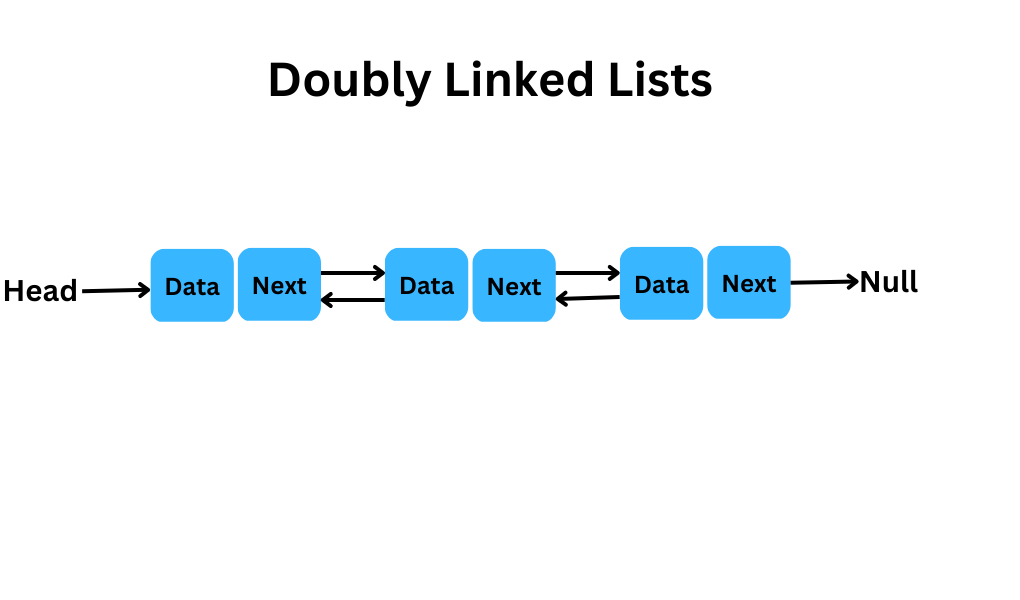
Circular Linked Lists
In circular linked lists, the last node points to the first node, forming a loop.
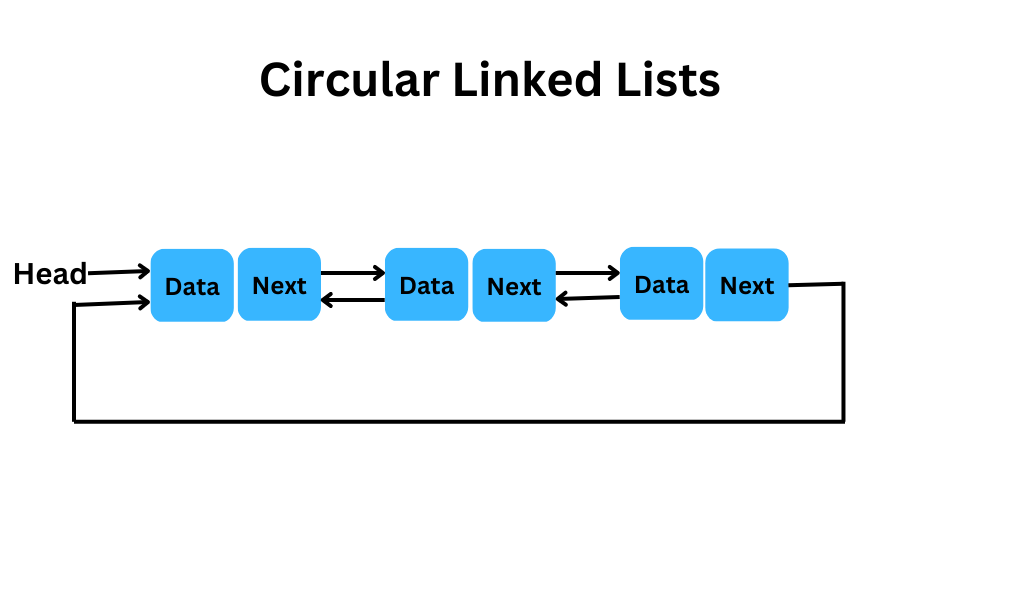
Advantages of Linked Lists :
1.Dynamic Memory Allocation
Linked lists allow for dynamic memory allocation, meaning that the amount of memory allocated can change during runtime. This makes them very useful in situations where the amount of data is unknown.
2.Erase Insert And Deletion
Insertion and deletion operations are very easy in linked lists. Unlike arrays, there is no need to shift elements around when an item is inserted or deleted.
3.Memories Efficiency
Linked lists use memory more efficiently than arrays. Arrays need to be allocated in contiguous memory locations, which can lead to wasted space. Linked lists, on the other hand, can use non-contiguous memory locations.
Disadvantages of Linked Lists
1.Random Access
Linked lists do not support random access. This means that to access a specific item in the list, you need to traverse the list from the beginning.
2.Memories Overhead
Linked lists have a higher memory overhead than arrays. Each node in the list requires additional memory for the pointer to the next node.
Real-World Applications of Linked Lists
- Text editors: Linked lists are used to store the text of a document and provide efficient insertion and deletion of characters.
- Music players: Linked lists are used to create playlists and provide fast access to the next song.
- Web browsers: Linked lists are used to store the history of visited websites and provide fast navigation between pages.
- Operating systems: Linked lists are used to manage processes and provide efficient memory allocation.
Conclusion
Linked lists are a powerful data structure that can simplify your programming projects. They are dynamic, easy to manipulate, and memory efficient. However, they do have some drawbacks, such as lack of random access and higher memory overhead.
If you want to become a proficient programmer, you should definitely learn about linked lists. They are a fundamental data structure that is widely used in many programming languages and applications.
FAQs
Q1.Can linked lists have loops?
A1. Yes, circular linked lists have a loop.
Q2. What is the difference between singly linked lists and doubly linked lists?
A2. Singly linked lists have each node pointing to the next node, while doubly linked lists have each node pointing to both the next and the previous nodes.
Q3. Can linked lists have duplicate nodes?
A3. Yes, linked lists can have duplicate nodes.
Q4. What is the memory efficiency of linked lists compared to arrays?
A4. Linked lists use memory more efficiently than arrays.
Related Topics
- Array
- Stacks
- Queue
- Linked List
- Tree
- Graph
- Heaps
- Hashing
- Singly Linked List
- Doubly Linked List
- Circular Linked List
- Linked List Operations
- Linked List Traversal
- Linked List Insertion
- Linked List Deletion
- Linked List Sorting
- Linked List Searching
- Merge two Linked Lists
- Reverse Linked List
- Linked List Implementation
- Linked List vs. Array
- Sparse Linked List
- Linked List Applications