Table of contents
Introduction
Queue are data structures that store data in a particular order, allowing new elements to be added to the end of the queue and existing elements to be removed from the front. Queues have various applications in computer science, including operating system scheduling, computer networks, simulation and modeling, and traffic management systems.
In this article, we’ll delve into the details of the queue data structure, how it works, its types, operations, advantages, and disadvantages, and its applications in different domains.
What is a Queue?
A queue is a linear data structure that follows the First-In-First-Out (FIFO) principle. In other words, the element that is added first is the one that will be removed first. It is like a queue of people waiting in line to buy tickets, where the first person to arrive is the first to get served.
A queue consists of two ends – front and rear. The front end is where the removal of elements takes place, and the rear end is where new elements are added. The process of adding elements to the rear end is called enqueue, and the process of removing elements from the front end is called dequeue.
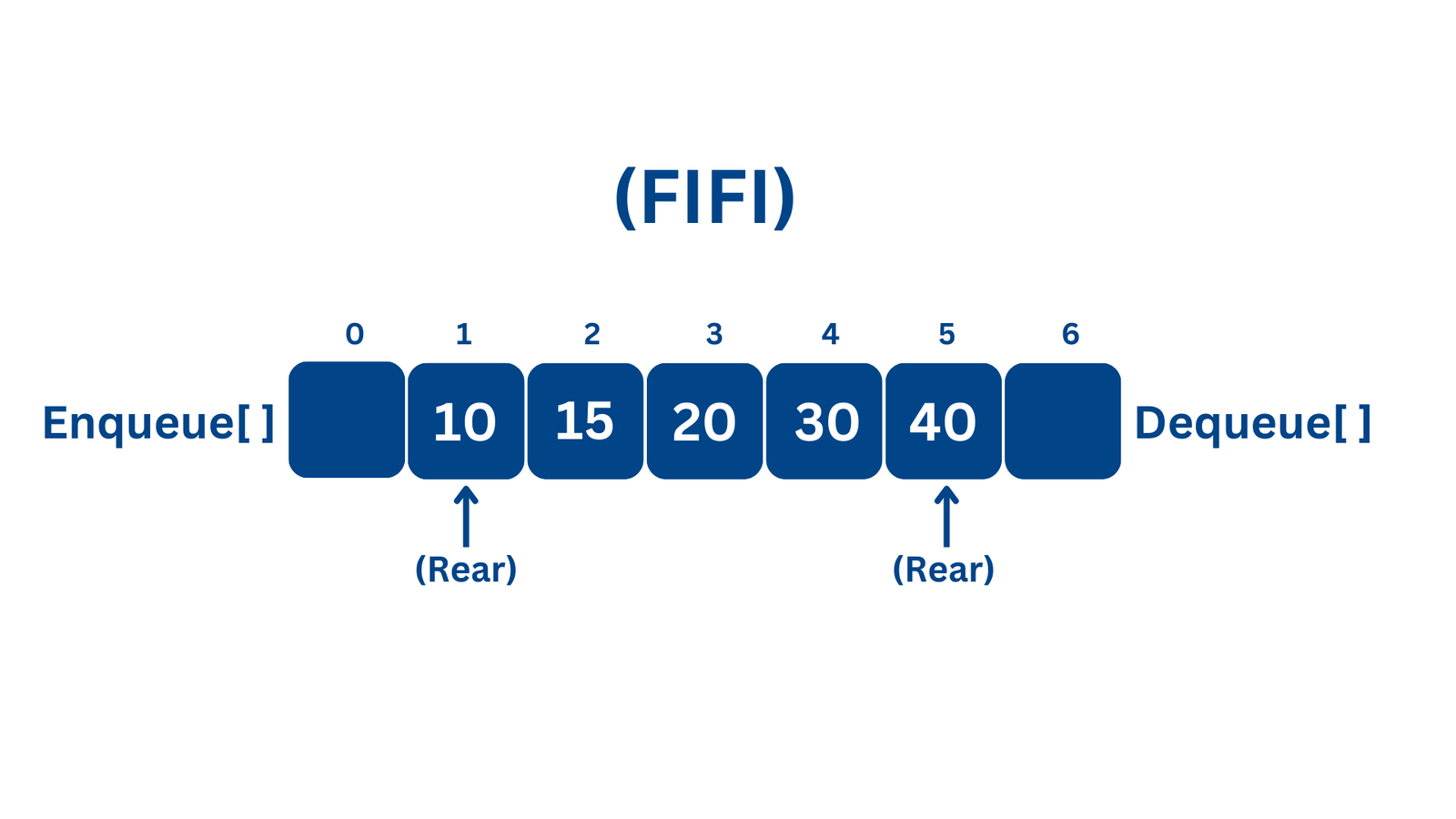
How Does a Queue Work?
A queue works by adding new elements to the rear end and removing elements from the front end. When a new element is added to the rear end, it becomes the last element in the queue. Similarly, when an element is removed from the front end, the next element in the queue becomes the new front.
Queues follow the FIFO principle, which means that the element that is added first is the one that will be removed first. This principle ensures that the elements in the queue are processed in the same order in which they were added.
Types of Queues
Queues can be classified into three main types based on their properties and operations:
Linear Queue
A linear queue is a simple type of queue where elements are added at one end and removed from the other. It has a fixed size, and once it’s full, no more elements can be added. Linear queues follow the FIFO principle and have two pointers – front and rear.
Circular Queue
A circular queue is atype of queue where the last element is connected to the first element, forming a circular structure. Circular queues also follow the FIFO principle, but they can continue to add elements even if the queue is full by overwriting the oldest element.
Priority Queue
A priority queue is a type of queue where elements are added with a priority value, and the element with the highest priority is removed first. Priority queues are commonly used in operating systems to schedule tasks and in computer networks to prioritize network traffic.
Queue Operations
Queues have several operations that can be performed on them to manage the elements. Here are the main operations of queues:
Enqueue
Enqueue is the process of adding a new element to the rear end of the queue. The element is added to the next available slot in the queue, and the rear pointer is updated to point to the new last element.
Dequeue
Dequeue is the process of removing the element from the front end of the queue. The element is removed, and the front pointer is updated to point to the next element in the queue.
Peek
Peek is the process of returning the value of the first element in the queue without removing it.
IsEmpty
IsEmpty is a Boolean operation that checks whether the queue is empty or not. If the queue is empty, it returns true; otherwise, it returns false.
IsFull
IsFull is a Boolean operation that checks whether the queue is full or not. If the queue is full, it returns true; otherwise, it returns false.
Advantages and Disadvantages of Queues
Like any other data structure, queues have their own advantages and disadvantages. Here are some of the advantages of using queues:
- Queues are easy to implement and understand.
- They are efficient for storing and accessing elements in a specific order.
- Queues are suitable for applications that require processing elements in a FIFO manner.
However, there are also some disadvantages of using queues:
- Queues have a fixed size, which means they can’t accommodate more elements than their size.
- Adding and removing elements from the middle of the queue is not efficient and requires shifting all the elements.
Applications of Queues
Queues have various applications in computer science, including:
Operating System Scheduling
In an operating system, queues are used to schedule processes and allocate resources. The processes are added to the ready queue, and the scheduler selects the next process to run based on its priority and other criteria.
Computer Networks
Queues are also used in computer networks to manage network traffic. The packets are added to the queue, and the router processes them based on their priority and the congestion level of the network.
Simulation and Modeling
Queues are commonly used in simulation and modeling to simulate real-life scenarios. For example, a queue can be used to simulate the waiting time at a supermarket checkout counter.
Traffic Management Systems
Queues are used in traffic management systems to manage traffic flow and avoid congestion. The traffic lights are programmed to switch based on the number of cars waiting in the queue.
Comparison with Other Data Structures
Queues are not the only data structure used in computer science. Here’s a comparison of queues with other data structures:
Stack
A stack is a data structure that follows the Last-In-First-Out (LIFO) principle, where the last element added is the first element to be removed. Stacks are commonly used in programming languages for function calls and memory allocation.
Linked List
A linked list is a data structure that consists of a sequence of nodes, where each node contains a pointer to the next node. Linked lists can be used to implement queues, but they require additional memory overhead.
Arrays
Arrays are a simple data structure that stores elements in contiguous memory locations. It can be used to implement queues, but they have a fixed size and can be inefficient when adding or removing elements from the middle of the queue.
Conclusion
Queues are a fundamental data structure in computer science that are used to manage elements in a specific order. They follow the First-In-First-Out (FIFO) principle and have several operations, such as enqueue, dequeue, peek, isEmpty, and isFull. There are several types of queues, including linear queues, circular queues, and priority queues, each with their own advantages and disadvantages. Queues have various applications, such as in operating system scheduling, computer networks, simulation and modeling, and traffic management systems. Finally, queues can be compared with other data structures, such as stacks, linked lists, and arrays.
FAQs
- What is the purpose of a queue data structure?
- A queue data structure is used to manage elements in a specific order, following the First-In-First-Out (FIFO) principle.
- What are the main operations of a queue?
- The main operations of a queue are enqueue, dequeue, peek, isEmpty, and isFull.
- What are the advantages of using queues?
- Queues are easy to implement and understand, efficient for storing and accessing elements in a specific order, and suitable for applications that require processing elements in a FIFO manner.
- What are the disadvantages of using queues?
- Queues have a fixed size, which means they can’t accommodate more elements than their size, and adding and removing elements from the middle of the queue is not efficient.
- What are some applications of queues?
- Queues have various applications in computer science, including operating system scheduling, computer networks, simulation and modeling, and traffic management systems
Related Topic
- Tree
- Binary Tree
- Heaps
- Hashing
- Circular Queues and its Applications
- Priority Queues: Definition and Usage
- Queue vs Stack: Differences and Similarities
- Queues in Operating Systems: Scheduling Algorithms
- Real-life Examples of Queues: Queuing Theory
- Queues in Networking: Packet Queuing
- Double-ended Queues: Deque Data Structure
- Queues in Multi-threaded Programming.