Table of contents
What is an Array?
An array is a collection of similar data elements that are stored in contiguous memory locations and accessed using a common name or index. In other words, an array is a collection of variables of the same data type that are accessed by a single name.
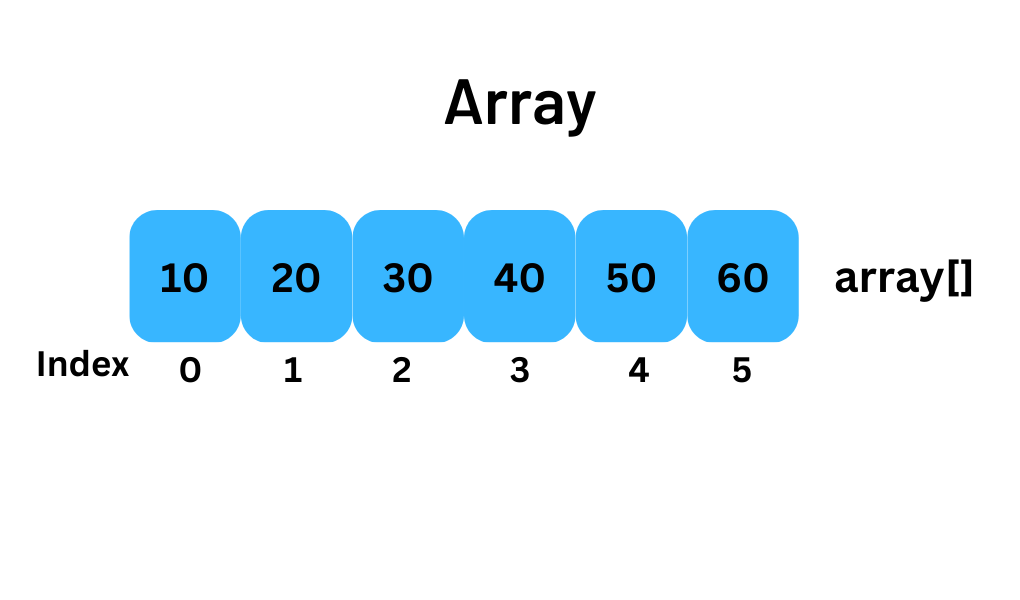
Types of Arrays
There are two types of arrays – one-dimensional arrays and multidimensional arrays.
One-dimensional Arrays
A one-dimensional array, also known as a vector, is a collection of elements of the same data type that are stored in contiguous memory locations. The elements are accessed using a single index, starting from 0.
Multidimensional Arrays
A multidimensional array is an array of arrays. It is a collection of elements of the same data type that are stored in contiguous memory locations. The elements are accessed using multiple indices, depending on the number of dimensions.
Advantages of Using Arrays
There are several advantages to using arrays:
- Arrays allow for efficient storage and retrieval of data.
- Arrays allow for easy manipulation of data.
- Arrays can be used to store large amounts of data.
Disadvantages of Using Arrays
There are also some disadvantages to using arrays:
- Arrays have a fixed size and cannot be easily resized.
- Arrays can be inefficient when inserting or deleting elements.
- Arrays can be difficult to manage and can lead to errors if not used correctly.
Using Arrays in Programming
Arrays are used extensively in programming. They can be used to store data, such as numbers, strings, and objects. They can also be used to represent matrices and other mathematical structures.
Declaring and Initializing Arrays
To declare an array, you must specify the data type of the elements and the size of the array. For example, to declare an array of integers with 5 elements, you would write:
int myArray[5];
To initialize an array, you can assign values to the elements at the time of declaration or later. For example, to initialize an array of integers with values 1, 2, 3, 4, and 5, you would write:
int myArray[] = {1, 2, 3, 4, 5};
Accessing Array Elements
To access an element in an array, you use the index of the element. For example, to access the third element in an array called myArray, you would write:
int x = myArray[2];
Conclusion
Arrays are one of the most fundamental data structures in computer science and programming. They are used to store and manipulate data efficiently and effectively. By understanding how arrays work and how to use them effectively, you can improve your programming skills and create more efficient and effective code.
FAQs
1.Can arrays be resized in programming?
In some programming languages, such as Python, arrays can be resized dynamically, but in other languages like C++, Its si is fixed at the time of declaration and cannot be easily resized.
2.What is the advantage of using multidimensional arrays?
Multidimensional arrays allow for the representation of more complex data structures, such as matrices and other mathematical structures.
3.What is the disadvantage of using arrays?
It have a fixed size and cannot be easily resized, which can be a disadvantage when dealing with large amounts of data or when the size of the data is not known in advance.
Related Topics
- Tree
- Binary Tree
- Heaps
- Hashing
- Arrays in Programming
- Multidimensional Arrays
- Dynamic Arrays
- Array Sorting Algorithms
- Array Searching Algorithms
- Array vs Linked List
- Array vs ArrayList
- Array vs Queue
- Array vs Stack
- Array vs Hash Table