Encapsulation and information hiding are fundamental concepts in object-oriented programming that promote code organization, security, and maintainability. Encapsulation involves bundling data and methods within a single unit, a class, to create self-contained and modular components. By hiding the implementation details of a class and exposing only essential interfaces, encapsulation protects sensitive data from unauthorized access and reduces the impact of changes on other parts of the program. Information hiding, closely related to encapsulation, emphasizes concealing the internal complexities of a class or module, allowing developers to interact with it through well-defined interfaces. Both concepts enable developers to manage code complexity, improve code reusability, and create robust and secure software systems. These principles play a crucial role in modern software development, enhancing software design and ensuring the effective implementation of object-oriented programming paradigms.
Encapsulation:
Encapsulation is one of the four fundamental principles of object-oriented programming (OOP) and is often associated with data hiding. It is the process of bundling data (attributes) and methods (functions) that operate on that data within a single unit, known as a class. The class serves as a blueprint for creating objects, which are instances of the class. Encapsulation allows for the organization of data and functionality into self-contained units, promoting code modularity, reusability, and security. Let’s delve deeper into the concept of encapsulation and its significance in object-oriented programming.
Principles of Encapsulation:
Encapsulation is guided by the following principles:
- Data Hiding: The internal representation of an object’s data is hidden from the outside world. This is achieved by declaring data members as private within the class. Outside entities can only access the data through public methods (getters and setters), ensuring data integrity and preventing unauthorized access.
- Abstraction: Encapsulation allows for the abstraction of complex data and functionality into simpler, higher-level units. This promotes a clear separation of concerns and reduces code complexity.
- Access Control: By defining access modifiers (public, private, protected), encapsulation enables fine-grained control over the visibility and accessibility of class members. This enhances security and prevents unintended modifications of sensitive data.
Benefits of Encapsulation:
Encapsulation offers several advantages in software development:
- Data Protection: Data hiding ensures that the internal state of an object is shielded from external manipulation, preventing data corruption and ensuring data integrity.
- Code Reusability: Encapsulated classes can be reused in various parts of a program or in different projects, reducing code duplication and promoting code reusability.
- Modularity: Encapsulation facilitates code organization into separate modules (classes), making the codebase easier to maintain and understand.
- Security: By restricting direct access to data, encapsulation enhances security, reducing the risk of unauthorized access and manipulation of sensitive information.
- Isolation of Changes: Encapsulation minimizes the impact of changes within a class to the rest of the program, promoting better code maintenance and reducing bugs.
Example
Encapsulation in C++:
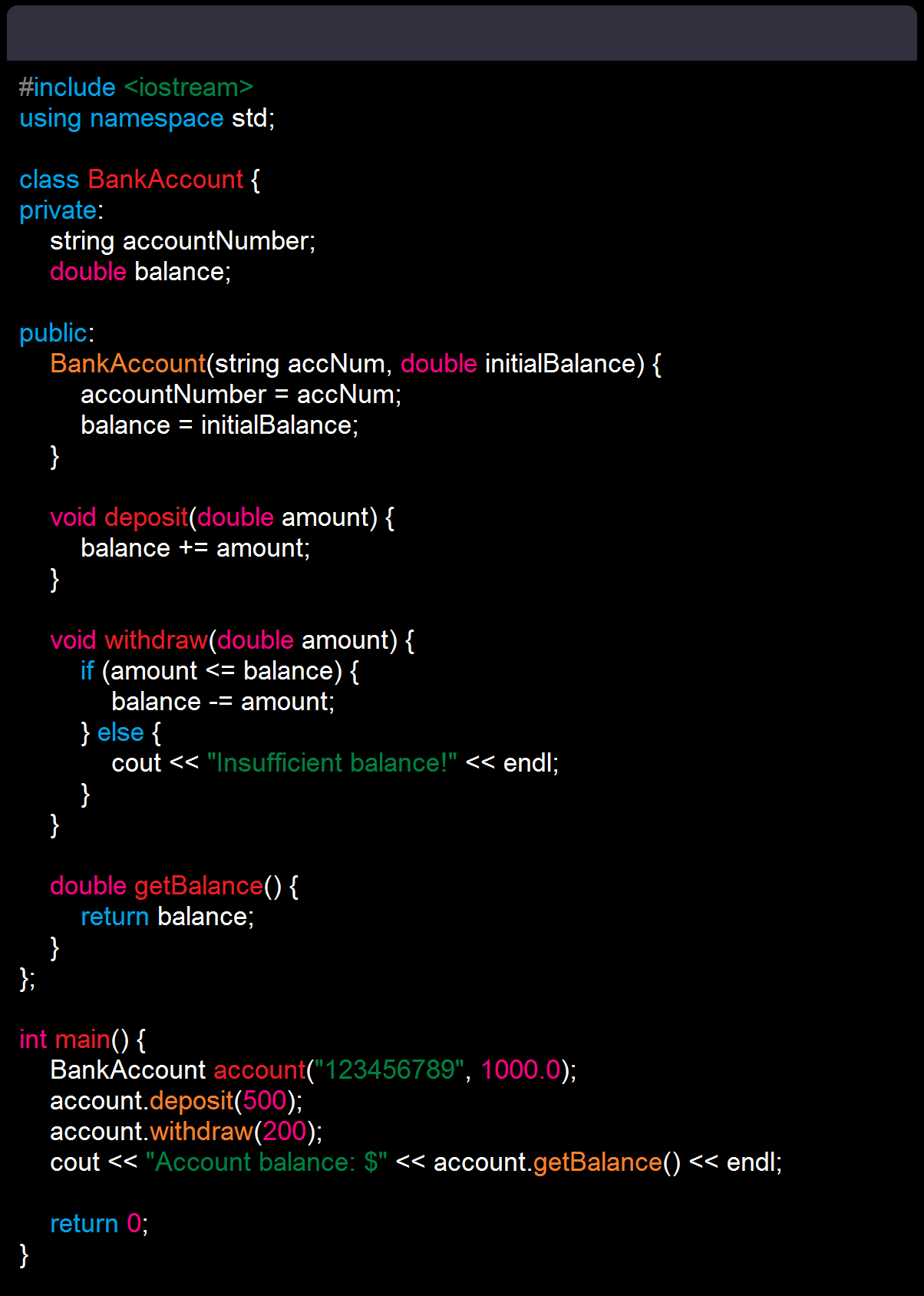
In this example, the BankAccount class encapsulates the account number and balance, allowing access to these attributes only through public methods (deposit, withdraw, getBalance). This ensures that the account data is protected and can be modified only through controlled operations.
Encapsulation in Inheritance and Polymorphism:
Encapsulation is closely related to other OOP concepts like inheritance and polymorphism. Inheritance allows classes to inherit properties and behavior from other classes, promoting code reuse. Polymorphism allows objects of different classes to be treated as objects of a common base class, enhancing code flexibility and extensibility.
Information Hiding:
Information hiding is a software design principle closely related to encapsulation. It emphasizes the need to hide implementation details of a class or module from the external world, exposing only essential interfaces. This approach helps manage the complexity of a system, promotes code modularity, and protects sensitive data. Information hiding plays a vital role in ensuring software security, maintainability, and flexibility. Let’s explore the concept of information hiding in detail and understand its importance in software development.
Principles of Information Hiding:
Information hiding is guided by the following principles:
- Hide Implementation Details: Internal data structures and algorithms of a class or module should be hidden from external entities. This is achieved by making the implementation details private and exposing only essential interfaces through public methods.
- Abstraction: Information hiding is a form of abstraction that allows developers to focus on high-level functionality while abstracting away the implementation complexities.
- Reduced Dependency: By hiding implementation details, developers can modify the internal workings of a class without affecting the external entities that use it. This reduces the dependency between different parts of the system.
Benefits of Information Hiding:
Information hiding provides several benefits in software development:
- Improved Security: Hiding sensitive data and implementation details reduces the risk of data breaches and unauthorized access, enhancing software security.
- Simplified Code Maintenance: Changes made to the internal implementation of a class have minimal impact on external code, making software maintenance easier.
- Code Reusability: Hiding implementation details allows developers to reuse code modules without worrying about their internal complexities.
- Enhanced Code Readability: By exposing only essential interfaces, information hiding makes code more readable and understandable.
Example
Information Hiding in Python:

In this example, the Customer class hides the name and email attributes from direct access by making them private (prefixed with double underscores). External entities can only access and modify these attributes using the public methods get_name and set_name.
Information Hiding in Libraries and APIs:
Information hiding is essential in library and API design. By exposing only essential functions and hiding the underlying implementation, developers can maintain backward compatibility and enhance the robustness of the library.
Conclusion
In conclusion, encapsulation and information hiding are essential principles in object-oriented programming, promoting code modularity, security, and maintainability. Encapsulation bundles data and methods within a class, while information hiding conceals the internal details of the class from external access. Together, these concepts enable developers to create robust and well-organized software systems, ensuring that changes to the internal implementation of classes do not affect other parts of the program. By providing clear interfaces for interaction and protecting sensitive data, encapsulation and information hiding enhance software design, reusability, and security, making them vital aspects of modern software development.
more related content on Principles of Programming Languages