Graph Traversal Algorithms
Graph traversal algorithms are techniques used to traverse or visit all the nodes in a graph systematically. They start at a particular node and explore the graph by following a set of rules or strategies. The two main graph traversal algorithms are DFS and BFS.
DFS Algorithm
DFS algorithm is a recursive algorithm that starts at a particular node and explores as far as possible along each branch before backtracking. It follows the ‘depth-first’ strategy, which means that it explores each node at the deepest possible level before backtracking.
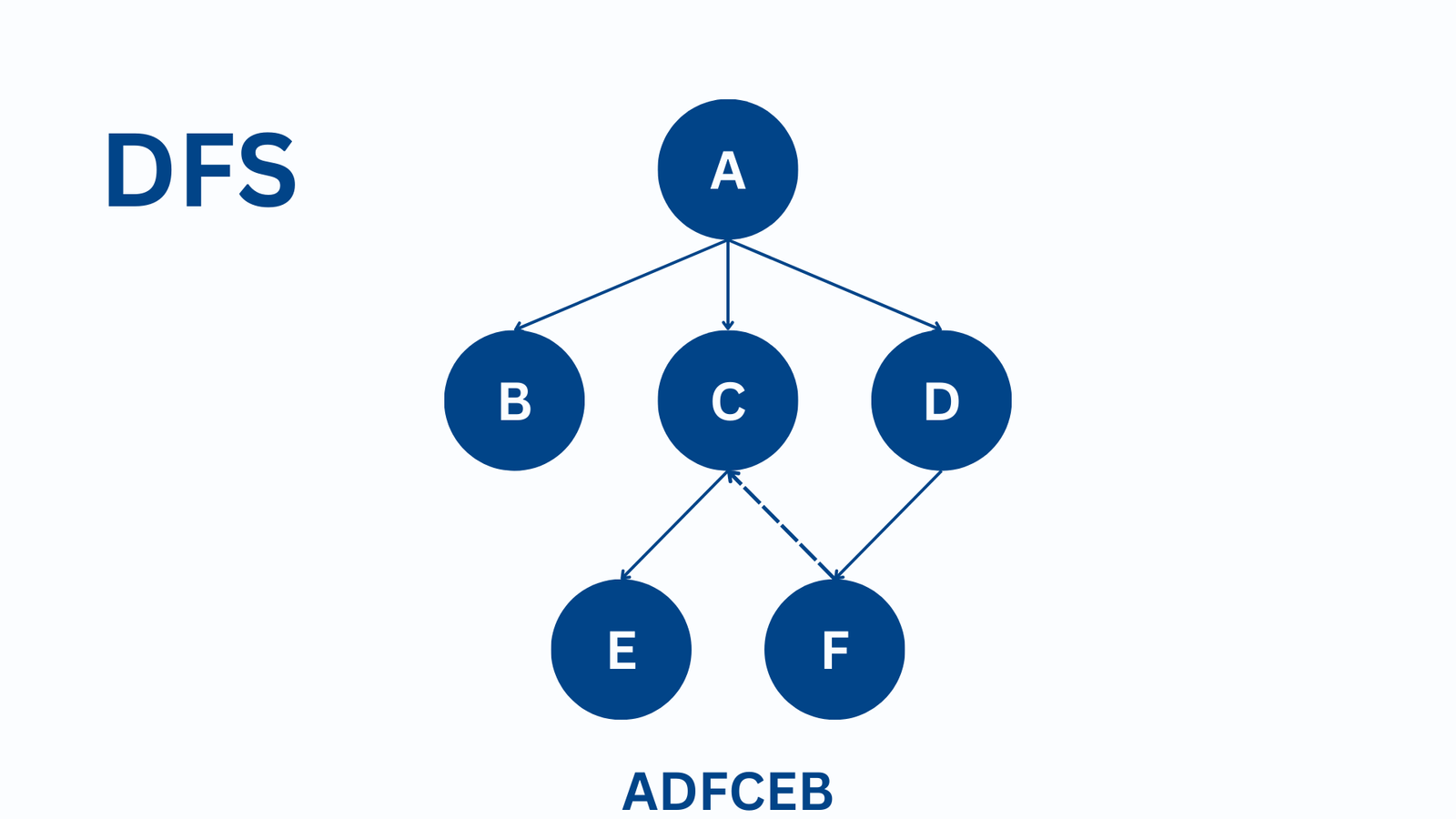
Recursive DFS Algorithm
The recursive DFS algorithm works by starting from a node and marking it as visited. It then recursively visits all its unvisited neighbors until there are no more unvisited neighbors. It then backtracks to the previous node and recursively visits the next unvisited neighbor until all nodes are visited.
Iterative DFS Algorithm
The iterative DFS algorithm works by using a stack data structure to keep track of the nodes to visit. It starts by pushing the starting node onto the stack and marking it as visited. It then repeatedly pops the top node from the stack, visits its unvisited neighbors, marks them as visited, and pushes them onto the stack. It continues until there are no more unvisited nodes.
DFS Algorithm:
- Create a stack to store visited nodes
- Push the starting node onto the stack
- While the stack is not empty, pop the top node and mark it as visited
- For each unvisited neighbor of the popped node, push it onto the stack
- Repeat steps 3-4 until the stack is empty or the desired node is found
DFS C++ Code:
void DFS(int start, vector<bool> &visited, vector<vector<int>> &graph) { stack<int> stk; stk.push(start); visited[start] = true; while (!stk.empty()) { int node = stk.top(); stk.pop(); cout << node << " "; for (auto neighbor : graph[node]) { if (!visited[neighbor]) { stk.push(neighbor); visited[neighbor] = true; } } } }
BFS Algorithm
BFS algorithm is a non-recursive algorithm that starts at the root node and explores all the neighbor nodes at the present depth before moving on to the nodes at the next depth level.
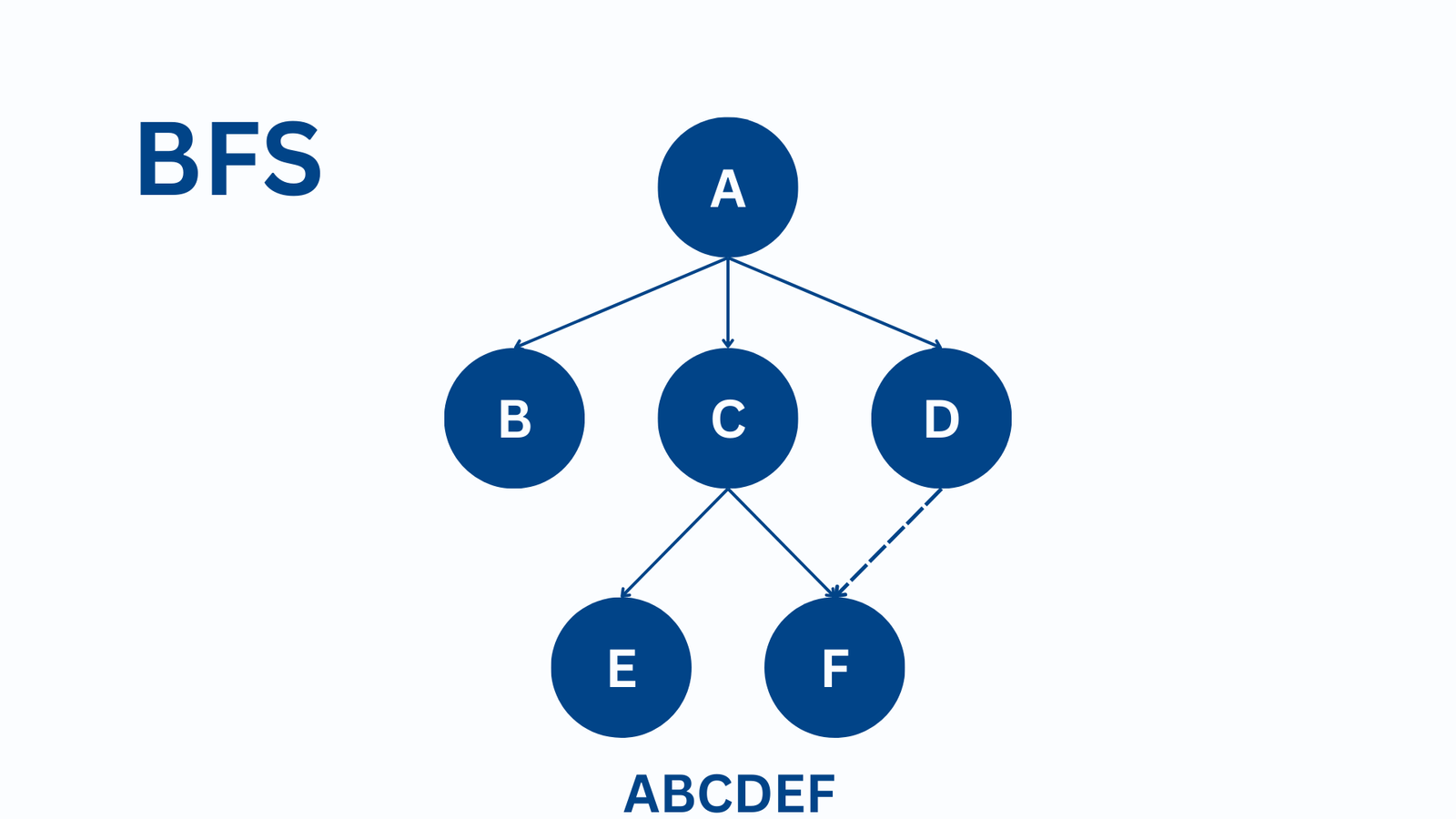
Iterative BFS Algorithm
The iterative BFS algorithm uses a queue data structure to keep track of the nodes to visit. It starts by enqueuing the root node and marking it as visited. It then repeatedly dequeues the front node from the queue, visits its unvisited neighbors, marks them as visited, and enqueues them to the back of the queue. It continues until there are no more unvisited nodes.
Bidirectional BFS Algorithm
The bidirectional BFS algorithm is a variant of the BFS algorithm that starts at both the source and destination nodes simultaneously. It then runs two BFS searches, one from the source node and one from the destination node. The algorithm terminates when the two BFS searches meet in the middle.
BFS Algorithm:
- Create a queue to store visited nodes
- Enqueue the starting node onto the queue
- While the queue is not empty, dequeue the front node and mark it as visited
- For each unvisited neighbor of the dequeued node, enqueue it onto the queue
- Repeat steps 3-4 until the queue is empty or the desired node is found
BFS C++ Code:
void BFS(int start, vector<bool> &visited, vector<vector<int>> &graph) { queue<int> q; q.push(start); visited[start] = true; while (!q.empty()) { int node = q.front(); q.pop(); cout << node << " "; for (auto neighbor : graph[node]) { if (!visited[neighbor]) { q.push(neighbor); visited[neighbor] = true; } } } }
Graph Search and Pathfinding Algorithms
Graph search algorithms are used to find a particular node in a graph or to determine whether a path exists between two nodes. Pathfinding algorithms are used to find the shortest path between two nodes in a graph. DFS and BFS algorithms can be used as graph search and pathfinding algorithms. However, there are other more efficient algorithms, such as Dijkstra’s algorithm and A* algorithm.
DFS Algorithm for Graph Search
DFS algorithm can be used as a graph search algorithm to determine whether a path exists between two nodes. It works by starting from the source node and recursively visiting all its unvisited neighbors until it finds the destination node or there are no more unvisited nodes.
BFS Algorithm for Graph Search
BFS algorithm can also be used as a graph search algorithm to determine whether a path exists between two nodes. It works by starting from the source node and exploring all its neighbor nodes at the present depth level before moving on to the nodes at the next depth level. It terminates when it finds the destination node or there are no more unvisited nodes.
BFS Algorithm for Shortest Pathfinding
BFS algorithm can also be used as a pathfinding algorithm to find the shortest path between two nodes in an unweighted graph. It works by assigning a distance of 1 to all the neighbor nodes of the source node, a distance of 2 to all the neighbor nodes of the neighbor nodes, and so on. It terminates when it finds the destination node or there are no more unvisited nodes. The shortest path can then be reconstructed by backtracking from the destination node to the source node.
Dijkstra’s Algorithm
Dijkstra’s algorithm is a pathfinding algorithm used to find the shortest path between two nodes in a weighted graph. It works by assigning a tentative distance to each node and then repeatedly selecting the node with the smallest tentative distance and updating its neighboring nodes’ tentative distances. The algorithm terminates when it finds the destination node or there are no more unvisited nodes.
A Algorithm*
A* algorithm is a pathfinding algorithm that uses both heuristic and cost functions to find the shortest path between two nodes in a weighted graph. It combines the best features of BFS and Dijkstra’s algorithm by considering both the distance to the start node and the estimated distance to the end node.
Applications of DFS and BFS Algorithms
DFS and BFS algorithms have numerous applications in various fields, including:
Artificial Intelligence
DFS and BFS algorithms are widely used in artificial intelligence for game playing, decision-making, and knowledge representation.
Networking
DFS and BFS algorithms are used in networking to find the shortest path between two network nodes and to detect cycles in a network.
Social Media Analysis
DFS and BFS algorithms are used in social media analysis to detect communities, influencers, and information diffusion patterns.