Introduction
Recurrence relation is a fundamental concept in the field of computer science, specifically in the area of algorithms and data structures. It refers to a way of defining a sequence of numbers, where each term is defined in terms of one or more preceding terms. Recurrence relation in DAA (Design and Analysis of Algorithms) is a powerful tool for analyzing the performance of algorithms and determining their time complexity. It is used extensively in various fields of computer science, including artificial intelligence, machine learning, and data science.
Recurrence relation can be represented in several ways, including closed-form expressions, iterative equations, and recursive formulas. Each of these representations has its advantages and disadvantages, depending on the context and application. In this article, we will explore the different types of recurrence relations and their properties, along with practical examples and use cases.
Types of Recurrence Relations
There are several types of recurrence relations, including linear, homogeneous, non-homogeneous, and nonlinear recurrence relations. Let’s take a closer look at each of these types.
Linear Recurrence Relations
A linear recurrence relation is one in which each term is a linear combination of the previous terms. That is, if the sequence is denoted by a_n, then it can be expressed as:
a_n = c_1 a_{n-1} + c_2 a_{n-2} + … + c_k a_{n-k}
where c_1, c_2, …, c_k are constants. Linear recurrence relations are widely used in DAA, as they can be easily solved using techniques such as the characteristic equation method and the matrix exponentiation method.
Homogeneous Recurrence Relations
A homogeneous recurrence relation is one in which the right-hand side of the recurrence equation is zero. That is, if the sequence is denoted by a_n, then it can be expressed as:
a_n = c_1 a_{n-1} + c_2 a_{n-2} + … + c_k a_{n-k}
where c_1, c_2, …, c_k are constants, and a_0, a_1, …, a_{k-1} are the initial terms of the sequence. Homogeneous recurrence relations are particularly useful in analyzing the time complexity of recursive algorithms, such as quicksort and merge sort.
Non-Homogeneous Recurrence Relations
A non-homogeneous recurrence relation is one in which the right-hand side of the recurrence equation is nonzero. That is, if the sequence is denoted by a_n, then it can be expressed as:
a_n = c_1 a_{n-1} + c_2 a_{n-2} + … + c_k a_{n-k} + f(n)
where c_1, c_2, …, c_k are constants, and f(n) is a non-constant function. Non-homogeneous recurrence relations are more difficult to solve than homogeneous ones, as they require the use of specific techniques such as the method of undetermined coefficients or the variation of parameters method.
Nonlinear Recurrence Relations
A nonlinear recurrence relation is one in which the recurrence equation involves one or more nonlinear functions of the previous terms. That is, if the sequence is denoted by a_n, then it can be expressed as:
a_n = f(a_{n-1}, a_{n-2}, …, a_{n-k})
Solving Recurrence Relations
Now that we have a basic understanding of the different types of recurrence relations, let’s dive into how we can solve them. There are several techniques for solving recurrence relations, including the characteristic equation method, the matrix exponentiation method, the method of undetermined coefficients, and the variation of parameters method. Let’s take a closer look at each of these techniques.
Practical Applications of Recurrence Relation in DAA
Recurrence relation is a powerful tool for analyzing the performance of algorithms and determining their time complexity. It is used extensively in various fields of computer science, including artificial intelligence, machine learning, and data science. Here are some practical applications of recurrence relation in DAA.
Sorting Algorithms
Sorting algorithms are a classic example of the use of recurrence relation in DAA. The time complexity of most sorting algorithms, such as bubble sort, insertion sort, and selection sort, can be analyzed using recurrence relation. For example, the time complexity of merge sort, a popular sorting algorithm, can be expressed as:
T(n) = 2T(n/2) + O(n)
where T(n) is the time complexity of the algorithm for a sequence of length n, and O(n) represents the time complexity of merging two sorted sub-sequences of length n/2.
Dynamic Programming
Dynamic programming is a technique for solving complex optimization problems by breaking them down into smaller sub-problems and solving each sub-problem only once. It is widely used in various fields, including artificial intelligence, machine learning, and data science. Recurrence relation plays a crucial role in dynamic programming, as it allows for the efficient computation of the solutions to the sub-problems. The time complexity of most dynamic programming algorithms can be expressed using recurrence relation, which can then be solved using the characteristic equation method or the matrix exponentiation method.
Fibonacci Numbers
The Fibonacci sequence is a classic example of the use of recurrence relation in DAA. The sequence is defined by the recurrence relation:
F_n = F_{n-1} + F_{n-2}
where F_0 = 0 and F_1 = 1. The sequence has numerous applications in various fields, including mathematics, computer science, and finance. The time complexity of computing the nth Fibonacci number using the naive recursive algorithm is O(2^n), which is exponential. However, the time complexity can be reduced to O(n) using dynamic programming or matrix exponentiation methods.
Methods For Solving Recurrence
Characteristic Equation Method
The characteristic equation method is a technique for solving linear recurrence relations with constant coefficients. It involves finding the roots of the characteristic equation, which is obtained by substituting a_n = r^n into the recurrence relation, where r is a constant. The roots of the characteristic equation correspond to the homogeneous solution to the recurrence relation, while the particular solution is obtained using the method of undetermined coefficients or the variation of parameters method.
Matrix Exponentiation Method
The matrix exponentiation method is a technique for solving linear recurrence relations with constant coefficients using matrix algebra. The recurrence relation is converted into a matrix equation, and the solution is obtained by computing the nth power of the matrix. The time complexity of this method is O(k^3 log n), where k is the size of the matrix.
Method of Undetermined Coefficients
The method of undetermined coefficients is a technique for finding a particular solution to a non-homogeneous recurrence relation. It involves guessing a solution based on the form of the non-homogeneous term and determining the coefficients by substitution into the recurrence relation.
Variation of Parameters Method
The variation of parameters method is another technique for finding a particular solution to a non-homogeneous recurrence relation. It involves finding a general solution to the homogeneous recurrence relation, then using the method of undetermined coefficients to find a particular solution. The general solution and the particular solution are then combined to obtain the general solution to the non-homogeneous recurrence relation.
Master Theorem
The master theorem is a formula for determining the time complexity of divide-and-conquer algorithms that can be expressed using a recurrence relation of the form T(n) = aT(n/b) + f(n), where a is the number of sub-problems, b is the size of the sub-problems, and f(n) is the time complexity of combining the sub-problems. The theorem has three cases, depending on the relative magnitudes of a, b, and f(n).
In conclusion, solving recurrence relation is an important step in analyzing the performance of algorithms and determining their time complexity. There are several methods for solving recurrence relation, including the characteristic equation method, the matrix exponentiation method, the method of undetermined coefficients, the variation of parameters method, and the master theorem. The choice of method depends on the form of the recurrence relation and the specific problem being solved.
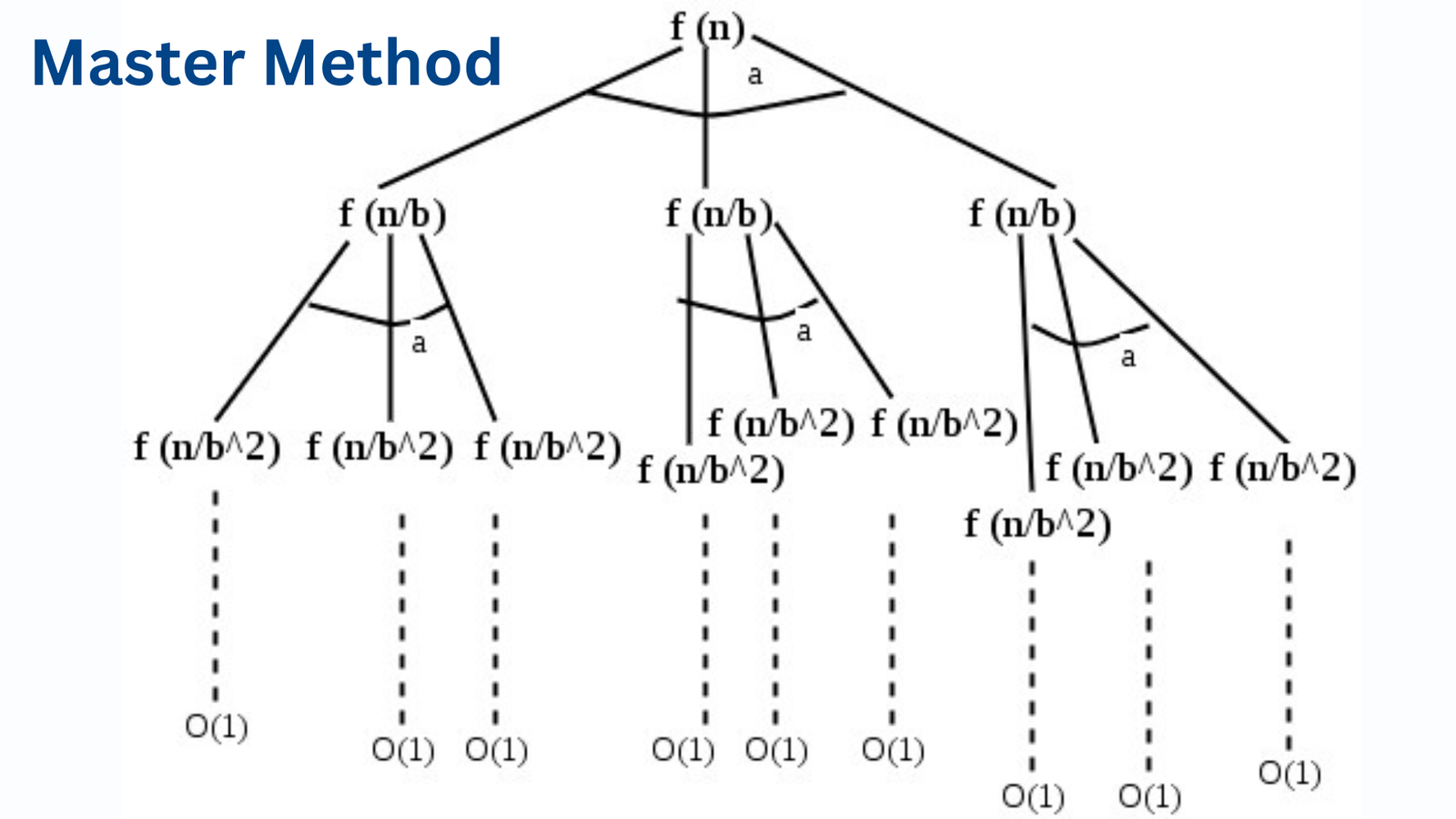
Conclusion
Recurrence relation is a powerful tool for analyzing the performance of algorithms and determining their time complexity. It is widely used in various fields of computer science, including artificial intelligence, machine learning, and data science. There are several techniques for solving recurrence relations, including the characteristic equation method, the matrix exponentiation method, the method of undetermined coefficients, and the variation of parameters method. Recurrence relation is a fundamental concept in DAA and is essential for understanding the time complexity of algorithms and developing efficient solutions to optimization problems.
FAQs
Here are some frequently asked questions about recurrence relation in DAA.
What is the difference between linear and non-linear recurrence relations?
Linear recurrence relations have the form a_n = c_1 a_{n-1} + c_2 a_{n-2} + … + c_k a_{n-k}, where c_1, c_2, …, c_k are constants. Non-linear recurrence relations do not have a similar form and can include higher powers of the sequence terms.
What is the characteristic equation method?
The characteristic equation method is a technique for solving linear recurrence relations with constant coefficients. It involves finding the roots of the characteristic equation and using them to obtain the general solution to the recurrence relation.
What is dynamic programming?
Dynamic programming is a technique for solving complex optimization problems by breaking them down into smaller sub-problems and solving each sub-problem only once. It is widely used in various fields, including artificial intelligence, machine learning, and data science.