JDBC (Java Database Connectivity)
Java Database Connectivity (JDBC) is a Java-based API (Application Programming Interface) that allows Java applications to interact with relational databases. JDBC provides a standard interface for connecting Java applications with databases and performing database operations such as querying, updating, inserting, and deleting data. It enables developers to write database-independent applications and facilitates the creation of dynamic, data-driven Java applications.
Steps Involved in JDBC Connectivity
JDBC connectivity involves several steps to establish a connection between a Java application and a relational database. These steps typically include:
Import JDBC Packages:
- Import the necessary JDBC packages into your Java program. Commonly used packages include
java.sql
for core JDBC function - ality and
javax.sql
for extended features.
import java.sql.*;
Load and Register the JDBC Driver:
- Load and register the appropriate JDBC driver for the database you intend to connect to. The driver is a platform-specific implementation provided by the database vendor.
Class.forName("com.mysql.cj.jdbc.Driver");
Establish a Connection:
- Use the
DriverManager.getConnection
method to establish a connection to the database. Provide the database URL, username, and password as parameters.
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/database", "username", "password");
Create a Statement:
- Create a
Statement
or aPreparedStatement
object to execute SQL queries against the database.
Statement statement = connection.createStatement();
Execute SQL Queries:
- Use the created statement to execute SQL queries and retrieve results.
ResultSet resultSet = statement.executeQuery("SELECT * FROM table");
Process Results:
- Process the results obtained from the executed queries. Iterate through the
ResultSet
to retrieve data.
while (resultSet.next()) { // Process data }
Close Resources:
- Close the
ResultSet
,Statement
, andConnection
objects to release resources and avoid memory leaks.
resultSet.close(); statement.close(); connection.close();
Four Types of JDBC Drivers
JDBC drivers facilitate the connection between Java applications and databases. There are four types of JDBC drivers, each with its characteristics and advantages. The types are often referred to as Type 1, Type 2, Type 3, and Type 4 drivers.
Type 1: JDBC-ODBC Bridge Driver (Bridge Driver):
- Description:
- The Type 1 driver translates JDBC calls into ODBC calls. It relies on an existing ODBC driver to communicate with the database.
- Also known as the “JDBC-ODBC Bridge” or “ODBC-JDBC Bridge.”
- Diagram:
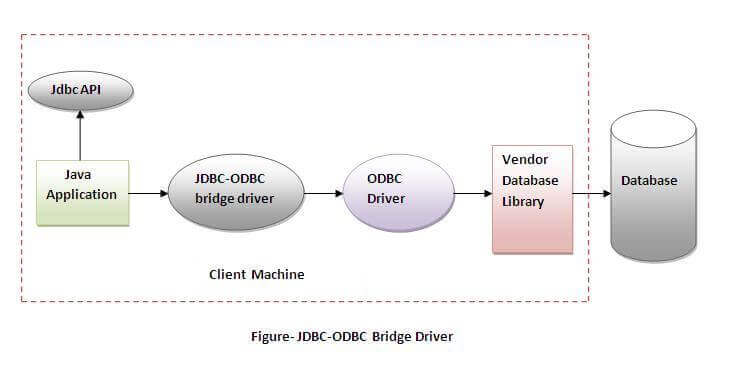
- Advantages:
- Easy to set up, especially if an ODBC driver is already available.
- Database independence at the application level.
- Disadvantages:
- Relies on the presence of an ODBC driver.
- Limited performance compared to other drivers.
Type 2: Native-API Driver (Partially Java Driver):
- Description:
- The Type 2 driver uses a database-specific native library to communicate with the database. It interacts with the database’s native API.
- Diagram:
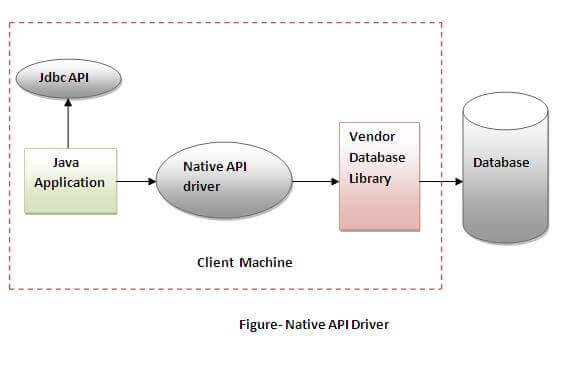
- Advantages:
- Better performance than Type 1.
- Database-specific optimizations are possible.
- Disadvantages:
- Requires platform-specific native code.
- Less portable than Type 1.
Type 3: Network Protocol Driver (Middleware Driver):
- Description:
- The Type 3 driver communicates with a middleware server, which, in turn, communicates with the database. It uses a specific network protocol.
- Diagram:
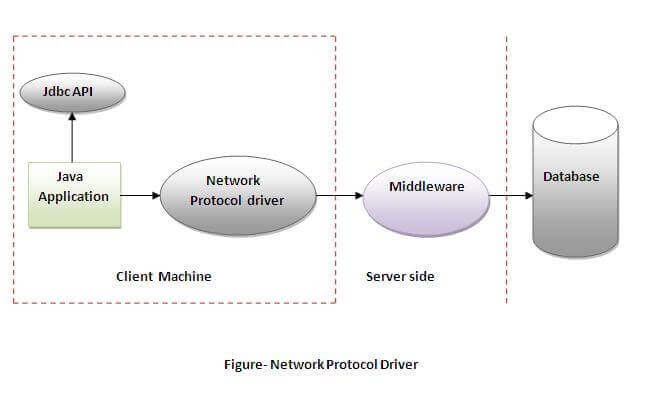
- Advantages:
- Database independence at the application level.
- Middleware provides additional services (security, load balancing).
- Disadvantages:
- Requires additional middleware.
- Performance may be affected by network latency.
Type 4: Thin Driver (Pure Java Driver):
- Description:
- The Type 4 driver is a pure Java implementation that communicates directly with the database using a database-specific protocol.
- Diagram:
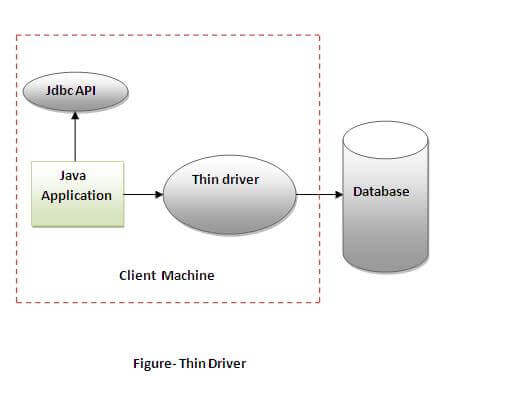
- Advantages:
- Platform-independent and does not require native code.
- Good performance and widely used in production environments.
- Disadvantages:
- Database-specific implementations required.
- Limited to databases with a Type 4 driver.
Conclusion
JDBC plays a crucial role in connecting Java applications to relational databases, enabling developers to create dynamic and data-driven applications. Understanding the steps involved in JDBC connectivity and the characteristics of the four types of JDBC drivers allows developers to make informed decisions when choosing the appropriate driver for their applications. Each driver type has its advantages and disadvantages, and the selection depends on factors such as performance requirements, database independence, and the need for platform-specific code. By mastering JDBC, developers can create efficient, scalable, and database-independent Java applications.
Add a Comment